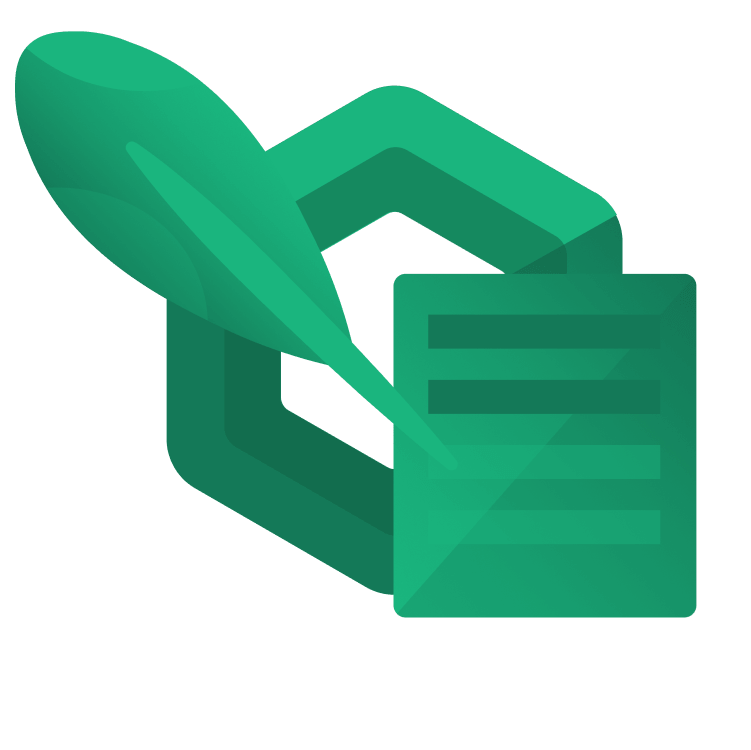
Graphics Using Jetpack Compose
Learn to create custom graphics using Jetpack Compose in Android with the convenient Canvas composable and the Paint object. By arjuna sky kok.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Graphics Using Jetpack Compose
25 mins
- Getting Started
- Creating Jetpack Compose Custom Graphics
- Using Declarative Graphics API
- Understanding Canvas
- Creating a Canvas
- Drawing on a Canvas With Jetpack Compose
- Using Modifier
- Creating Objects With Jetpack Compose
- Drawing Lines
- Drawing Circles
- Drawing Point Lines
- Drawing Arcs
- Drawing Complex Shapes: Blinky the Ghost
- Drawing the Ghost’s Feet
- Drawing the Ghost’s Body
- Drawing the Ghost’s Head
- Drawing the Ghost’s Eyes
- Drawing Text With Jetpack Compose
- Scaling, Translating and Rotating Objects With Jetpack Compose
- Scaling and Translating Objects
- Rotating Objects
- Where to Go From Here?
Rotating Objects
The Pacman game has a lives indicator, and adding one will make this complete. The indicator is the Pacman shape itself. So, if the indicator has three Pacman shapes, it means you have three lives. You already know how to draw Pacman. Now, you need to duplicate him. But that’s not enough. You also need to move the clones, make them smaller, and then rotate them.
Go into drawPacmanLives
, and add the following code:
scope.withTransform({
scale(0.4f)
translate(top=1200.dp.value, left=-1050.dp.value)
rotate(180f)
}) {
drawArc(
Color.Yellow,
45f,
270f,
true,
pacmanOffset,
Size(200.dp.value, 200.dp.value)
)
}
You’ve seen the drawArc
code before. It’s the code you used when you drew the original Pacman. withTransform
will scale, translate, and finally, rotate Pacman. rotate
accepts the angle argument in degrees.
Build the project and run the app. You’ll see another Pacman but smaller and in a different place:
Now, try to draw another Pacman beside this clone to indicate that you have two lives left in the game.
Where to Go From Here?
Download the final project by clicking Download Materials at the top or bottom of this tutorial.
You’ve learned about the Graphics API in Jetpack Compose. You learned how to set up Canvas and modify it using a modifier. You’ve drawn many shapes and transformed them. You also used the native Canvas to draw text on it.
But there are more things you haven’t explored, such as animation. You could move Pacman and make his mouth open to simulate eating the dots. Of course, you could give life to the ghost, make it flash blue, and have it chase Pacman.
Feel free to checkout this wonderful Getting Started with Jetpack Compose Animations tutorial followed by the more advanced Jetpack Compose Animations tutorial.
We hope you enjoyed this tutorial! If you have any comments or questions, please join the forum discussion below.