Moving the Queen
The Queen is the most powerful piece, so that's an excellent place to finish.
The Queen's movement is a combination of the Bishop and Rook; the base class has an array of directions for each piece. It would be helpful if you could combine the two.
In Queen.cs, replace MoveLocations
with the following:
public override List<Vector2Int> MoveLocations(Vector2Int gridPoint)
{
List<Vector2Int> locations = new List<Vector2Int>();
List<Vector2Int> directions = new List<Vector2Int>(BishopDirections);
directions.AddRange(RookDirections);
foreach (Vector2Int dir in directions)
{
for (int i = 1; i < 8; i++)
{
Vector2Int nextGridPoint = new Vector2Int(gridPoint.x + i * dir.x, gridPoint.y + i * dir.y);
locations.Add(nextGridPoint);
if (GameManager.instance.PieceAtGrid(nextGridPoint))
{
break;
}
}
}
return locations;
}
The only thing that's different here is that you're turning the direction array into a List
.
The advantage of the List
is that you can add the directions from the other array, making one List
with all of the directions. The rest of the method is the same as the Bishop code.
Hit play again, and get the pawns out of the way to make sure everything works.
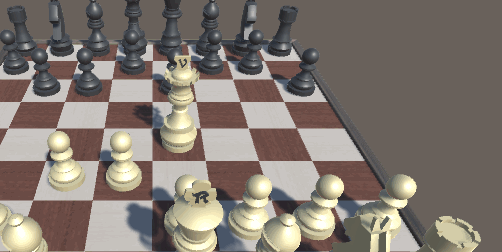
Where to Go From Here?
There are several things you can do at this point, like finish the movement for the King, Knight and Rook. If you're stuck at any point, check out the final project code in the project materials download.
There are a few special rules that are not implemented here, such as allowing a Pawn's first move to be two spaces instead of just one, castling and a few others.
The general pattern is to add variables and methods to GameManager
to keep track of those situations and check if they're available when the piece is moving. If available, then add the appropriate locations in MoveLocations
for that piece.
There are also visual enhancements you can make. For example, the pieces can move smoothly to their target location or the camera can rotate to show the other player's view during their turn.
If you have any questions or comments, or just want to show off your cool 3D chess game, join the discussion below!