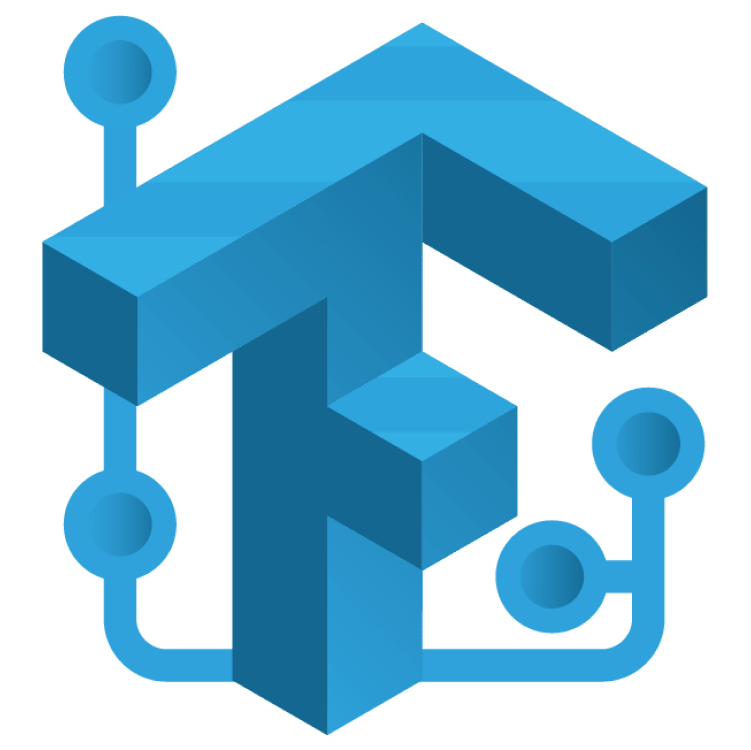
TensorFlow Lite Tutorial for Flutter: Image Classification
Learn how to use TensorFlow Lite in Flutter. Train your machine learning model with Teachable Machine and integrate the result into your Flutter mobile app. By Ken Lee.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
TensorFlow Lite Tutorial for Flutter: Image Classification
30 mins
- Getting Started
- Brief Introduction to Machine Learning
- What is Machine Learning
- Training a Model: How it Works
- Understanding Tensor and TensorFlow Prediction
- Installing TensorFlow Lite in Flutter
- Creating an Image Classifier
- Importing the Model to Flutter
- Loading Classification Labels
- Importing TensorFlow Lite Model
- Implementing TensorFlow Prediction
- Pre-Processing Image Data
- Running the Prediction
- Post-Processing the Output Result
- Using the Classifier
- Picking an Image From the Device
- Initializing the Classifier
- Analyzing Images Using the Classifier
- Where to Go from Here?
Using the Classifier
Now that it’s built, you’d probably like to understand how this app uses Classifier
to determine the name of the plant and display the results.
All the code from this section is already implemented in the starter project, so just read and enjoy!
Picking an Image From the Device
Your machine needs a photo to analyze, and you need to allow users to capture a photo they took from either the camera or photo album.
This is how you do that:
void _onPickPhoto(ImageSource source) async {
// #1
final pickedFile = await picker.pickImage(source: source);
// #2
if (pickedFile == null) {
return;
}
// #3
final imageFile = File(pickedFile.path);
// #4
setState(() {
_selectedImageFile = imageFile;
});
}
And here’s how the code above works:
- Pick an image from the image source, either the camera or photo album.
- Implement handling in case the user decides to cancel.
- Wrap the selected file path with a
File
object. - Change the state of
_selectedImageFile
to display the photo.
Initializing the Classifier
Here’s the code used to initialize the classifier:
@override
void initState() {
super.initState();
// #1
_loadClassifier();
}
Future _loadClassifier() async {
debugPrint(
'Start loading of Classifier with '
'labels at $_labelsFileName, '
'model at $_modelFileName',
);
// #2
final classifier = await Classifier.loadWith(
labelsFileName: _labelsFileName,
modelFileName: _modelFileName,
);
// #3
_classifier = classifier;
}
Here’s how that works:
- Run asynchronous loading of the classifier instance. Note that the project doesn’t contain enough error-handling code for production, so the app may crash if something goes wrong.
- Call
loadWith(...)
with the file paths for your label and model files. - Save the instance to the widget’s state property.
Analyzing Images Using the Classifier
Look at the following code in PlantRecogniser
at lib/widget/plant_recogniser.dart.
void _analyzeImage(File image) async {
// #1
final image = img.decodeImage(image.readAsBytesSync())!;
// #2
final resultCategory = await _classifier.predict(image);
// #3
final result = resultCategory.score >= 0.8
? _ResultStatus.found
: _ResultStatus.notFound;
// #4
setState(() {
_resultStatus = result;
_plantLabel = resultCategory.label;
_accuracy = resultCategory.score * 100;
});
}
The above logic works like this:
- Get the image from the file input.
- Use
Classifier
to predict the best category. - Define the result of the prediction. If the score is too low, less than 80%, it treats the result as Not Found.
- Change the state of the data responsible for the result display. Convert the score to a percentage by multiplying it by 100.
You then invoked this method in _onPickPhoto()
after imageFile = File(pickedFile.path);
:
void _onPickPhoto(ImageSource source) async {
...
final imageFile = File(pickedFile.path);
_analyzeImage(imageFile);
}
Here’s the effect when everything is set:
Where to Go from Here?
Great job. You made it to the end of this TensorFlow and Flutter tutorial!
Download the completed project by clicking Download Materials at the top or bottom of the tutorial.
You learned how to use TensorFlow Lite in a Flutter application, and if you weren’t familiar with machine learning already — you are now.
You also have the basic skills needed to implement a machine-learning solution that can solve problems and answer questions for your users.
If you’re interested in exploring classification more deeply, check out our Machine Learning: End-to-end Classification tutorial to learn more.
Also, if you’d like to learn more about normalizing data for a model from TensorFlow’s documentation, take a look at TensorFlow’s normalization and quantization parameters.
Furthermore, if you need a more robust solution than you can create with Teachable Machine, you could use a different learning framework such as Keras or PyTorch to create machine-learning models. These frameworks are more difficult to learn than Teachable Machine; however, they provide more features.
Tensorflow and Teachable Machine have quite a bit more to offer, and here is the best place to go to learn:
We hope you have enjoyed this tutorial. If you have any questions or comments, please join the forum discussion below!