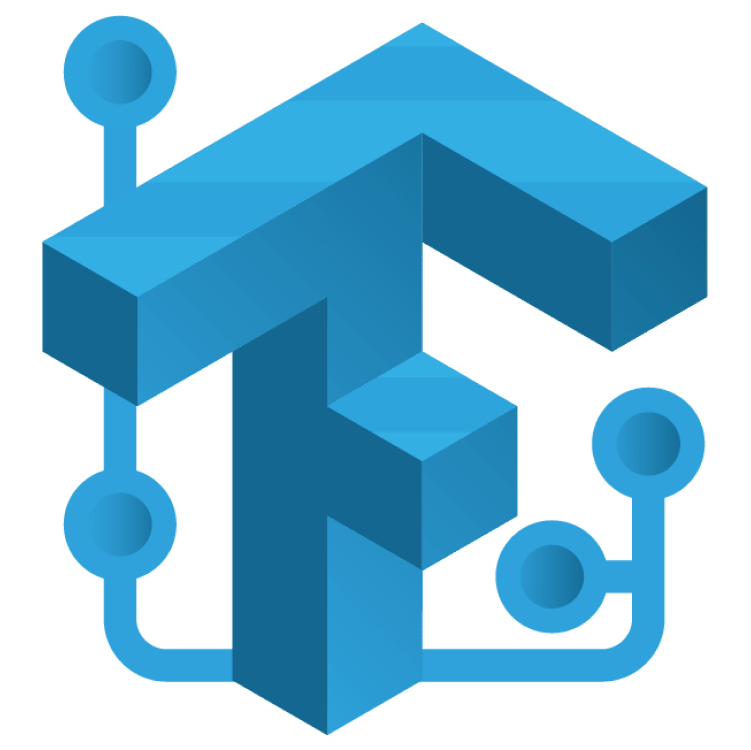
TensorFlow Lite Tutorial for Flutter: Image Classification
Learn how to use TensorFlow Lite in Flutter. Train your machine learning model with Teachable Machine and integrate the result into your Flutter mobile app. By Ken Lee.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
TensorFlow Lite Tutorial for Flutter: Image Classification
30 mins
- Getting Started
- Brief Introduction to Machine Learning
- What is Machine Learning
- Training a Model: How it Works
- Understanding Tensor and TensorFlow Prediction
- Installing TensorFlow Lite in Flutter
- Creating an Image Classifier
- Importing the Model to Flutter
- Loading Classification Labels
- Importing TensorFlow Lite Model
- Implementing TensorFlow Prediction
- Pre-Processing Image Data
- Running the Prediction
- Post-Processing the Output Result
- Using the Classifier
- Picking an Image From the Device
- Initializing the Classifier
- Analyzing Images Using the Classifier
- Where to Go from Here?
Machine learning is one of the hottest technologies of the last decade. You may not even realize it’s everywhere.
Applications such as augmented reality, self-driving vehicles, chatbots, computer vision, social media, among others, have adopted machine learning technology to solve problems.
The good news is that numerous machine-learning resources and frameworks are available to the public. Two of those are TensorFlow and Teachable Machine.
In this Flutter tutorial, you’ll develop an application called Plant Recognizer that uses machine learning to recognize plants simply by looking at photos of them. You’ll accomplish this by using the Teachable Machine platform, TensorFlow Lite, and a Flutter package named tflite_flutter
.
By the end of this tutorial, you’ll learn how to:
- Use machine learning in a mobile app.
- Train a model using Teachable Machine.
- Integrate and use TensorFlow Lite with the tflite_flutter package.
- Build a mobile app to recognize plants by image.
TensorFlow is a popular machine-learning library for developers who want to build learning models for their apps. TensorFlow Lite is a mobile version of TensorFlow for deploying models on mobile devices. And Teachable Machine is a beginner-friendly platform for training machine learning models.
Getting Started
Download the project by clicking Download Materials at the top or bottom of the tutorial and extract it to a suitable location.
After decompressing, you’ll see the following folders:
- final: contains code for the completed project.
- samples: has sample images you can use to train your model.
- samples-test: houses samples you can use to test the app after it’s completed.
- starter: the starter project. You’ll work with this in the tutorial.
Open the starter project in VS Code. Note that you can use Android Studio, but you’ll have to adapt the instructions on your own.
VS Code should prompt you to get dependencies — click the button to get them. You can also run flutter pub get
from the terminal to get the dependencies.
Build and run after installing the dependencies. You should see the following screen:
The project already allows you to pick an image from the camera or media library. Tap Pick from gallery to select a photo.
As you can see, the app doesn’t recognize images. You’ll use TensorFlow Lite to solve that in the next sections. But first, here’s an optional, high-level overview of machine learning to give you a gist of what you’ll do.
Brief Introduction to Machine Learning
This section is optional because the starter project contains a trained model model_unquant.tflite and classification labels in the labels.txt file.
If you’d prefer to dive into TensorFlow Lite integration, feel free to skip to Installing TensorFlow Lite.
What is Machine Learning
In this Flutter tutorial, you need to solve a classification problem: plant recognition. In a traditional approach, you’d define rules to determine which images belong to which class.
The rules would be based on patterns such as that of a sunflower, which has a large circle in the center, or a rose, which is somewhat like a paper ball. It goes like the following:
The traditional approach has several problems:
- There are many rules to set when there are many classification labels.
- It’s subjective.
- Some rules are hard to determine by the program. For example, the rule “like a paper ball” can’t be determined by a program because a computer doesn’t know what a paper ball looks like.
Machine learning offers another way to solve the problem. Instead of you defining the rules, the machine defines its own rules based on input data you provide:
The machine learns from the data, and that’s why this approach is called machine learning.
Before you continue, here’s some terminology you may need to know:
-
Training: The process by which the computer learns data and derives rules.
Building a Model with Teachable Machine
Preparing the Dataset
Training the Model
Now, you’ll learn how to train a model with Teachable Machine. The steps you’ll follow include:
Your first step is to prepare your dataset — the project needs plant photos. So your dataset is a collection of plants you want to recognize.
In a production-ready app, you’d want to collect as many varieties of a plant and as many plants as possible for your dataset to ensure higher accuracy. You’d do that by using your phone camera to take pictures of these plants or download images from various online sources that offer free datasets such as this one from Kaggle.
However, this tutorial uses plants from the samples folder, so you can also use it as a starting point.
Whichever one you use, it’s important to keep the number of samples for each label at similar levels to avoid introducing bias to the model.
Next, you’ll learn how to train the model using Teachable Machine.
First, go to https://teachablemachine.withgoogle.com and click Get Started to open the training tool:
Then select Image Project:
Choose Standard Image Model, because you’re not training a model to run on a microcontroller:
Once you’ve entered the training tool, add the classes and edit the labels of each class, as shown below:
Next, add your training samples by clicking Upload under each class. Then, drag the folder of the appropriate plant type from the samples folder to the Choose images from your files … panel.
After you’ve added all the training samples, click Train Model to train the model:
After the training completes, test the model with other plant images.
Use the images in the samples-test folder, like so:
Finally, export the model by clicking Export Model on the Preview panel. A dialog displays:
In the dialog, choose TensorFlow Lite. That’s because your target platform is mobile.
Next, select Floating point conversion type for the best predictive performance. Then, click Download my model to convert and download the model.
It may take several minutes to complete the model conversion process. Once it’s done, the model file will automatically download to your system.
After you have the model file converted_tflite.zip in hand, decompress it and copy labels.txt and model_unquant.tflite to the ./assets folder in your starter project.
Here’s what each of those files contains:
-
Model: The object created from training. It comprises the algorithm used to solve the AI problem and the learned rules.
- Preparing the dataset
- Training the model
- Exporting the model
Building a Model with Teachable Machine
Now, you’ll learn how to train a model with Teachable Machine. The steps you’ll follow include:
Your first step is to prepare your dataset — the project needs plant photos. So your dataset is a collection of plants you want to recognize.
Preparing the Dataset
In a production-ready app, you’d want to collect as many varieties of a plant and as many plants as possible for your dataset to ensure higher accuracy. You’d do that by using your phone camera to take pictures of these plants or download images from various online sources that offer free datasets such as this one from Kaggle.
However, this tutorial uses plants from the samples folder, so you can also use it as a starting point.
Whichever one you use, it’s important to keep the number of samples for each label at similar levels to avoid introducing bias to the model.
Training the Model
Next, you’ll learn how to train the model using Teachable Machine.
First, go to https://teachablemachine.withgoogle.com and click Get Started to open the training tool:
Then select Image Project:
Choose Standard Image Model, because you’re not training a model to run on a microcontroller:
Once you’ve entered the training tool, add the classes and edit the labels of each class, as shown below:
Next, add your training samples by clicking Upload under each class. Then, drag the folder of the appropriate plant type from the samples folder to the Choose images from your files … panel.
After you’ve added all the training samples, click Train Model to train the model:
After the training completes, test the model with other plant images.
Use the images in the samples-test folder, like so:
Finally, export the model by clicking Export Model on the Preview panel. A dialog displays:
In the dialog, choose TensorFlow Lite. That’s because your target platform is mobile.
Next, select Floating point conversion type for the best predictive performance. Then, click Download my model to convert and download the model.
It may take several minutes to complete the model conversion process. Once it’s done, the model file will automatically download to your system.
After you have the model file converted_tflite.zip in hand, decompress it and copy labels.txt and model_unquant.tflite to the ./assets folder in your starter project.
Here’s what each of those files contains:
- Preparing the dataset
- Training the model
- Exporting the model
- labels.txt: The label of each class.
- model_unquant.tflite: The trained machine learning model for plant recognition.