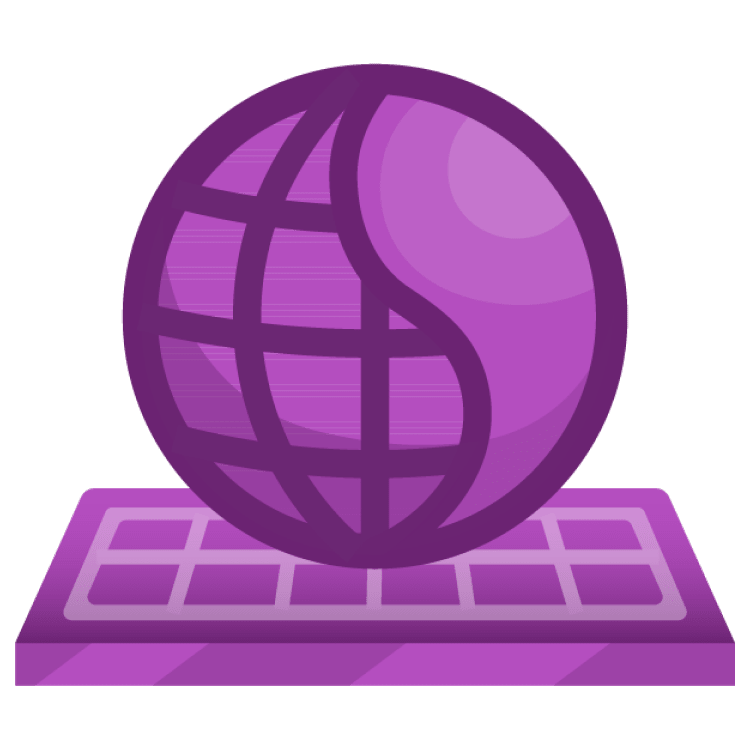
Unreal Engine 5 Blueprints Tutorial
In this Unreal Engine 5 Blueprints tutorial, you’ll learn how to use Blueprints to create a player character, set up inputs and make an item disappear when the player touches it. By Ricardo Santos.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Unreal Engine 5 Blueprints Tutorial
25 mins
- Getting Started
- Creating the Player
- Attaching a Camera
- Representing the Player
- Implementing the Player
- Creating a Game Mode
- World Settings
- Placing the Player Start
- Setting Up Inputs
- Axis Value and Input Scale
- Axis and Action Mappings
- Creating Movement Mappings
- Moving the Player
- Using Variables
- Getting the Player Direction
- Adding the Offset
- Frame Rate Independence
- Actor Collisions
- Enabling Collision
- Creating an Item
- Setting the Collision Response
- Handling Collision
- Placing the Item
- Where to Go From Here?
As of version 4, the Unreal Engine editor comes equipped with the Blueprints visual scripting system in addition to traditional C++ support. This system’s main purpose is to provide a friendlier programming interface than good ol’ C++, allowing the fast creation of prototypes or even allowing designers to customize game element behavior.
The Blueprint scripting system’s main feature is the ability to create code by creating boxes that represent functions and clicking and dragging wires to create behavior and define how the logic should work.
In this tutorial, you’ll use Blueprints to:
- Set up a top-down camera.
- Create a player-controlled actor with basic movement.
- Set up player inputs.
- Create an item that disappears when the player touches it.
This tutorial also makes basic use of vectors. If you’re not familiar with vectors, check out this article on vectors at gamedev.net
This tutorial also makes basic use of vectors. If you’re not familiar with vectors, check out this article on vectors at gamedev.net
Getting Started
Download the starter project by clicking the Download Materials button at either the top or bottom of this tutorial and unzip it. To open the project, go to the starter project folder and open BananaCollector.uproject.
You’ll see the scene below. This is where the player will move around and collect the items.
You’ll find the project files organized in folders:
- All/Content/BananaCollector/Blueprints: Contains the Blueprints of the tutorial.
- All/Content/BananaCollector/Maps: Contains the level of the project.
- All/Content/BananaCollector/Materials: Contains the game object shaders.
- All/Content/BananaCollector/Meshes: Contains the mesh files of all the project game objects.
- All/Content/BananaCollector/Textures: Contains the textures of the project game objects.
Use the button highlighted in red above to keep the Content Drawer fixed on the Editor window. If the button doesn’t appear, the Content Drawer is already fixed.
Creating the Player
In the Content Drawer, navigate to the Blueprints folder. Click the Add button and select Blueprint Class.
The actor must be able to receive inputs from the player, so select the Pawn class from the pop-up window and name it BP_Player.
To know more about the differences between the Character and the Pawn, read this article by Epic Games.
To know more about the differences between the Character and the Pawn, read this article by Epic Games.
Attaching a Camera
A camera is the player’s method of looking into the world. You’ll create a camera that looks down toward the player.
In the Content Drawer, double-click on BP_Player to open it in the Blueprint editor.
To create a camera, go to the Components panel. Click Add Component and select Camera.
For the camera to be in a top-down view, you must place it above the player. With the camera component selected, go to the Viewport tab.
Activate the move manipulator by pressing the W key and then move it to (-1100, 0, 2000). Alternatively, type the coordinates into the Location fields. They’re under the Transform section in the Details panel.
If you’ve lost sight of the camera, press the F key to focus on it.
Next, activate the rotation manipulator by pressing the E key. Rotate the camera down to -60 degrees on the Y-axis.
The final camera properties should be as shown below.
Representing the Player
A red cube will represent the player, so you’ll need to use a Static Mesh component to display it.
First, deselect the Camera component by left-clicking an empty space in the Components panel. If you don’t do this, the next added component will be a child of the Camera component.
Click Add and select Static Mesh.
To display the red cube, select the Static Mesh component and then go to the Details tab. Click the drop-down located to the right of Static Mesh and select SM_Cube.
This is what you should see (type F inside the Viewport to focus on this if you don’t see it):
Now, it’s time to spawn the player Pawn. Click Compile and return to the main editor.
Implementing the Player
Before the player can control the Pawn, you need to specify two things:
- The Pawn class the player will control
- Where the Pawn will spawn
You accomplish the first by creating a new Game Mode class.
Creating a Game Mode
A Game Mode is a class that controls how a player enters the game. For example, in a multiplayer game, you would use Game Mode to determine where each player spawns. More importantly, the Game Mode determines which Pawn the player will use.
Go to the Content Drawer and ensure you’re in the Blueprints folder. Click the Add New button and select Blueprint Class.
From the pop-up window, select Game Mode Base and name it GM_Tutorial.
Now, you need to specify which Pawn class will be the default. Double-click on GM_Tutorial to open it.
Go to the Details panel and look under the Classes section. Click the drop-down for Default Pawn Class and select BP_Player.
Click Compile and close the Blueprint editor. Before using your new Game Mode, the level needs to know which Game Mode to use. Specify this in World Settings.
World Settings
Each level has its settings. Access the settings by selecting menu Window ▸ World Settings. Alternatively, go to the toolbar and select Settings ▸ World Settings.
A new World Settings tab will open next to the Details tab. From here, click the drop-down for GameMode Override and select GM_Tutorial.
You’ll now see that the classes have changed to the ones selected in GM_Tutorial.
Finally, you need to specify where the player will spawn. You do this by placing a Player Start actor into the level.