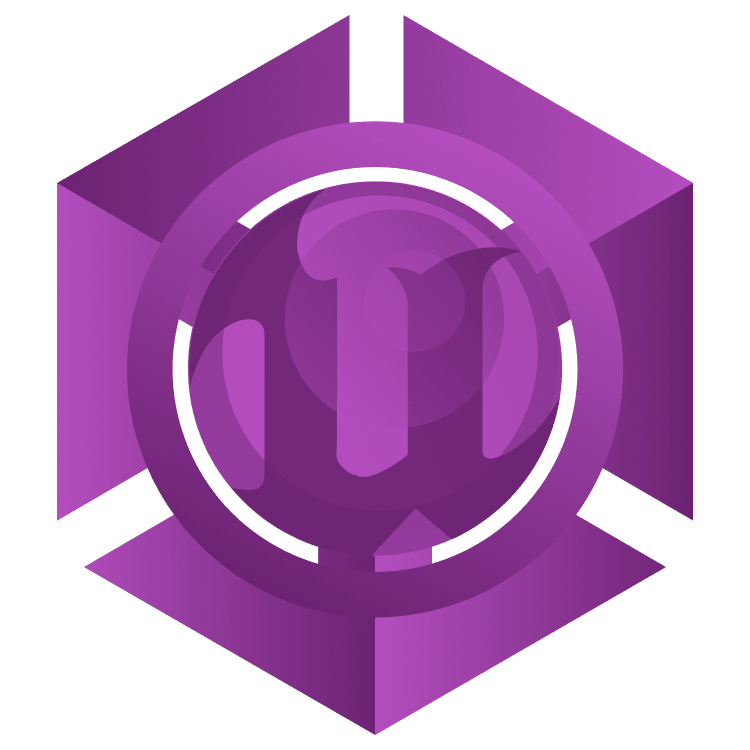
Getting Started with Unreal Engine for Unity Developers
This comprehensive tutorial introduces developers to Unreal Engine, highlighting its differences from Unity and providing a detailed guide on getting started. It covers various aspects, including the user interface, materials, blueprint visual scripting and exporting projects, making it an invaluable resource for those transitioning to Unreal Engine for game development. By Ricardo Santos.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Getting Started with Unreal Engine for Unity Developers
25 mins
Differing Architecture
The architecture of Unreal Engine projects is one of the main ways it differs from Unity. Unity allows you to ignore the need for a Game Manager class or mix the player controller along with game logic. There’s nothing — except your experience with best practices — stopping you from doing that.
In contrast, Unreal forces you to divide your game into these classes and have a good game architecture. To illustrate that, open World Settings and look at the topmost dropdown boxes.
These are all the elements that an Unreal Engine project must have, but the most important of them are:
- GameMode: A class responsible for applying the game rules and defining the game elements.
- Default Pawn: A class that defines the player in-game avatar. In Unreal, the pawn class defines this.
- HUD Class: A class that handles the game interface in a level.
- Player Controller: The interface between the player and the pawn, it transmits the player commands to the pawn.
This is one of the game architecture elements from before. You must define all these classes for your project. If you don’t define one of these classes, Unreal will provides a default one for you. This isn’t necessarily bad. The default Player Controller class can handle a game’s basic needs, for example. Consider making implementations only if your project requires special functionalities.
The default Pawn class is the sample character provided by Unreal Engine, which you can personalize it according to your project’s needs. You can change the character model, for instance, and create your own pawn. Also, this pawn is the one that will be spawned in the level at the initial player position.
The HUD class is blank by default because the default project has no interface. You can edit or create a new object to create your HUD and place it here.
The other classes aren’t changed often, so this tutorial won’t cover them.
Adding Materials
At this point, you have a pawn walking on a blank space. You might have rearranged some of the level models. You might have added some objects from the Quickly add to the project button, but the level remains blank. To change that, you can add some of the default assets that Unreal has already packed for you as Starter Content.
To see this content, first click the Content Drawer button on the bottom left of the Unreal Editor window. Next, click the Dock in Layout button to keep the drawer open as the Content Browser tab.
You should see the Materials folder in the Content Browser under All/Content/StarterContent. Click this folder to see the materials available from the starter content. Experiment by dragging and dropping the materials to the level elements to get a feel of how they work.
Unreal materials are like Unity’s materials, and produce all sorts of effects. For starters, find the M_Glass material in the Content Browser and drag and drop it on one of the level elements, such as a platform.
The platform has become transparent, with diffraction and refraction effects like you’d expect glass to behave. Enter Play mode, and you can walk on and around this glass monolith.
Now, things get more interesting. Search the starter contents materials and find the M_Tech_Hex_Tile_Pulse material. This is an animated material that changes color over time. Apply it on the ground like you applied the glass to that level platform.
Well, that doesn’t seem to be correct. The color changes and the material behaves as it should, but the size doesn’t seem right. At least the process to fix this can provide pretty good insight into how to work with materials!
First, copy the material into Content/LevelPrototyping/Materials to preserve the original asset. Remember to change the original material in the level for the copied one. Do that by dragging and dropping the newly created material in the level floor.
Double-click the copied material, and the Material Editor window appears.
This window works a lot like Unity’s Shader Graph — and like the Blueprint Editor window, which you’ll see in the next section. Notice how some nodes sit inside little gray boxes; this is how Unreal graphs display code comments.
To change how the texture displays in the level, navigate to the BumpOffset block. Find the TexCoord[0] node and edit the properties UTiling and VTiling to 30. After that, click the Apply button.
Click save and see how it looks in the level. If you want, keep trying new values until you think the floor looks good!
Feel free to change other material parameters in the editor! For example, change the colors the material alternates between in the Emissive graph section. When you finish experimenting, enter Play mode. See how the emissive color reflects on the default character and other level elements!
Using the Blueprint Visual Scripting Language
In the lower level, Unreal Engine uses C++ as the programming language. This a more complex language than Unity’s C# or Godot’s GDScript, even with the simplifications that Epic Games implemented. For C++ to get closer to a script language, Unreal added functionalities such as garbage collection and automatic memory management. In fact, unless you’re talking about the lower-level implementations in Unreal, it’s more correct to call the language Unreal C++.
To avoid requiring C++ knowledge, Unreal Engine provides the Blueprint scripting language, which is a visual scripting language that’s simple to learn and powerful for creating both prototypes and games.
In Unreal, blueprints roughly serve the same function as prefabs in Unity. They’re a container that embed the geometry, logic, animations, sounds, and other assets necessary for them to work. This is yet another way in which Unreal promotes modularity into projects.
To see how this works, double-click the BP_ThirdPersonCharacter Blueprint in the Content Browser under All/Content/ThirdPerson/Blueprints to open it.
The blueprint editor window should open and display the information about the Blueprint you see. The initial editor window shows the Blueprint nodes that cause the character to move around in the game level.
Looking at the node names, you can see they handle game inputs for camera movement and character movement. They also provide the input mapping for the character movement. Unreal handles user input in a particular way that can seem a little difficult at first.
To create an input, you must define an Input Action data asset. You can find the template project Input Actions in All/Content/ThirdPerson/Input/Actions. Double-click them to see their properties, each of which is accessible through event nodes in the Blueprint editor.
The key mapping is defined in the Input Mapping Context object named IMC_Default, present in the All/Content/ThirdPerson/Input folder. Double-click it to see its properties.
All input bindings are mapped in this data object, and you can change them as you like. The gameplay will then reflect this.
To define an input yourself, you’d need to create a new Input Action and then use the input context to bind them to a key or button. However, this whole system is too extensive of a subject to address here; if you have interest in this subject, you can read more in its Unreal Engine documentation page.
Another important part of the Blueprint editor window is the Viewport. This is where you can see the geometry that the Blueprint class renders in the game world. To access this view, click the Viewport tab on the top right of the event graph window.
You now see the game character in the central window, the Components section on the left side and the My Blueprint tab right below it. On the right, there’s the Details tab, which defines the object properties. To understand how this editor works, you’ll create a backpack for the game character.
In the Components tab, select the object Mesh(CharacterMesh0). The game character should appear outlined in the viewport, indicating that it’s selected.
Click the Add button, then search for the basic cube shape and name it Backpack.
With the backpack selected, go to the Details tab and set the Transform coordinates according to the figure below:
The final result should look like the figure below.
Click the Compile button on the screen top left and on the Save button. After a successful compile, the button icon should change from the question mark inside a yellow circle to a check mark inside a green circle.
Go back to the level viewport and enter play mode. The character should now have a rectangular backpack attached to its back. Kind of like the Death Stranding backpack design, right?
Notice that depending on how the character moves, the backpack doesn’t follow along. That’s because you added it as a child object of the character with constant coordinates, not as a part of the rigged character. In other words, the backpack stays in the hard-coded coordinate points — it doesn’t react to the character skeleton movement.