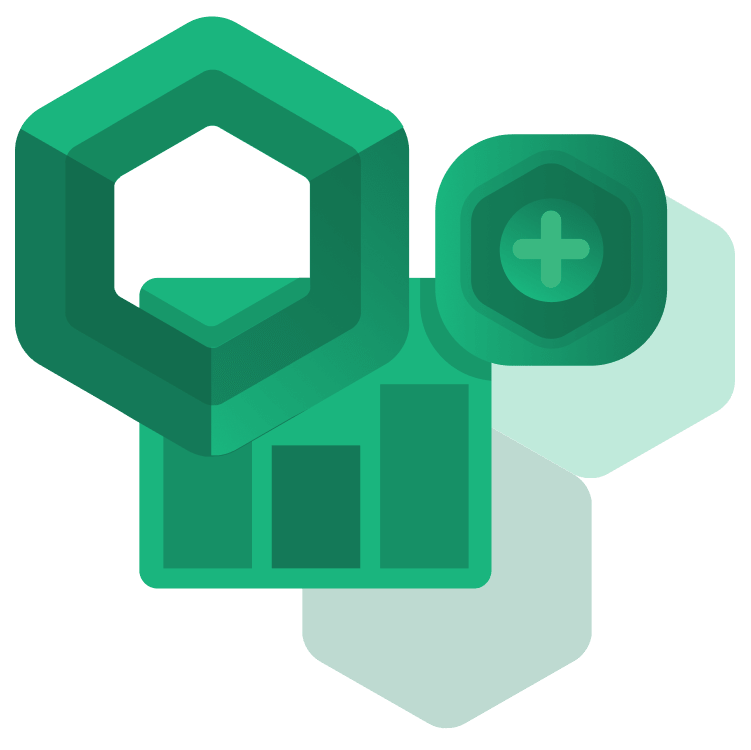
Jetpack Compose: Getting Started
In this Jetpack Compose Course, you’ll learn what Compose is, what its fundamental components are, and how they behave individually, and when composed together. Furthermore, you’ll learn how to build beautiful and reactive UI, with a lot of meaningful motion when it comes to animating custom components, input field state handling, error handling, and automatic state updates within an MVVM environment. By Ian Okumu.
Who is this for?
This course is designed for individuals looking to learn the basics of building declarative UIs in Android app development using Jetpack Compose.
Covered concepts
- Composable Functions
- Simple Composables
- Layouts
- Lists
- Actions & Handlers
- Modifiers
- State
- Recomposition
- Custom Composables
- Material Components
- Component Reusability
Part 1: Make a Simple Interface
In this episode, you will be able to understand the programming paradigms related to building user interfaces. We will focus on how the declarative UI development paradigm works and how it is different from the imperative UI development paradigm. You will learn how Jetpack Compose uses the declarative UI development paradigm to build user interfaces.
In this episode, you will understand what a composable function is and how you can create your first composable function to display some text. You will also get to learn how to use Jetpack Compose preview functions to view your composable function without having to run the application multiple times.
In this episode, you will learn how to build a simple layout using Jetpack Compose. You will learn how to use the Column
, Row
, Box
and Scaffold
composable functions to build a simple layout. You will also learn how to use the Modifier
parameter to modify the layout of the composable functions.
In this episode, you will learn how to leverage the power of modifiers to customize the appearance and behavior of your Composables. You will learn how to use the Modifier
class to customize the appearance and behavior of your Composables. You will also learn how to use the Modifier
class to add padding, add a background color, add a border, add a click handler to your composables.
In this session we will learn how to add a button to our Compose UI. We will also get a chance to customize the button’s appearance and behavior. Finally, we will look at how to handle user clicks on the button.
In this challenge, you will add several texts to different parts of the app. You will add three texts in a Column to our FoodDetails screen. You will also add extra text to the ProfileBar to indicate a welcome message. Finally create a FoodDetailMeasure Composable that will receive three parameters: icon, text and iconColor. This Composable will have an icon and text arranged horizontally.
Part 2: Layout Your App
In this session, you will learn how to use the Column and Row Composables to arrange Composables vertically and horizontally respectively. We will dive a little deeper into how to align items within a Column and Row
In this session, you will learn how to use the Spacer Composable to add space between Composables. You will also learn how to use the weight Modifier function to specify how much space an item should take up within a Column or Row Composable.
In this session, you will learn how to organize your code by extracting Composables into separate files. We will also discuss some important practices to follow when organizing your code.
In this session, you will learn how to add a TextField Composable to the app. You will also use different parameters available on the TextField Composable to customize its appearance.
Part 3: Jetpack Controls
In this session, you will learn how to customize the appearance of the TextField Composable. You will look at how to attach other Composables to the TextField Composable and even customize the colors within the TextField Composable.
In this session, you will learn how to display images in the app. You will also learn how to use the Image
Composable to display images in the app.
We will get to also customize the appearance of the image using the modifier
parameter.
In this session, you will learn how to use the Checkbox and Radio Button Composables to allow users to select one or more options from a list of options.
In this challenge, you will add trailing and leading icons to TextFields.
In this session, you will learn how to use a Scaffold Layout. You will also learn how to add a TopAppBar and a BottomAppBar to a Scaffold Layout. We will also get to learn how to add a Floating Action Button to a Scaffold Layout.
In this episode, you will learn how to utilize the power of lazy layouts to display lists of data in your app.
In this episode, you will learn how to manage state in Jetpack Compose. We will get to define what state is and how it affects rendering on the UI. We will also learn how to use the remember
API to manage state in our apps.
In this session, we will look at how Jetpack Compose facilitates recomposition of the UI. We will look at what happens when a state changes and how the UI is recomposed. We will also look at how to control recomposition within our Composables.