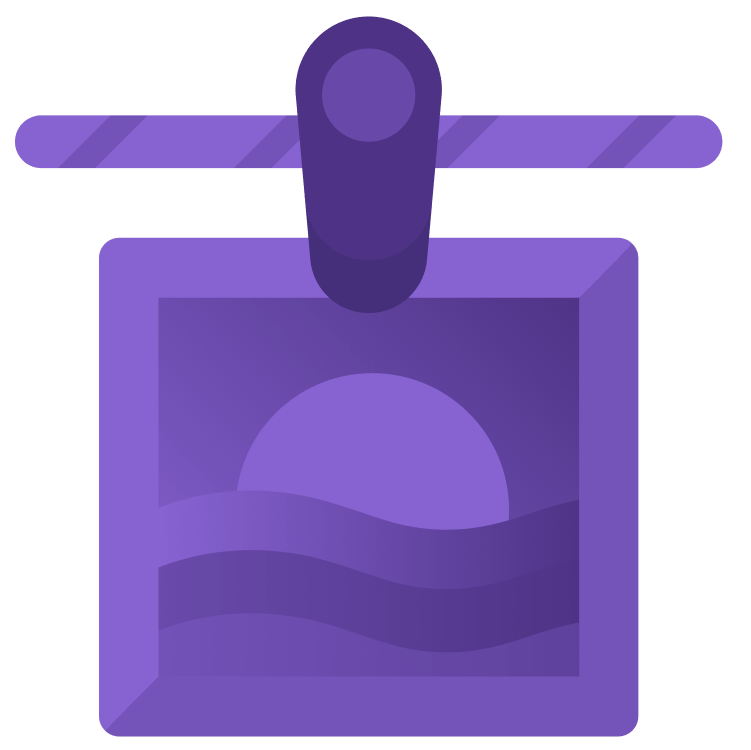
What’s New With PhotosPicker in iOS 16
PhotosPicker in iOS 16 has a robust set of features for users to view and select assets, such as images and videos. By Felipe Laso-Marsetti.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
What’s New With PhotosPicker in iOS 16
15 mins
Filter Options
Depending on the device you’re testing with, you may have noticed that the picker isn’t limiting selections to only photos — it includes everything from videos to screen recordings. You’ll need to use some of the available filter options to specify what types of selections the user can make.
Now, look at PHPickerFilter
. It lets you choose from several filters:
- bursts: Burst-style photos (ones with several photos taken at a high speed).
- cinematicVideos: More modern, cinematic-style videos with focus transitions and shallow depth of field.
- depthEffectPhotos: Photos taken with the depth effect.
- images: Images, including Live Photos.
- livePhotos: Live Photos exclusively.
- panoramas: Panoramic photos.
- screenRecordings: Videos of screen recordings.
- screenshots: Screenshots (usually taken by hitting the power and volume up buttons on devices with Face ID).
- slomoVideos: Slow-motion videos.
- timelapseVideos: Time-lapse videos.
- videos: Videos.
A couple helpful static functions also create a PHPickerFilter
for you:
-
all(of: [PHPickerFilter])
: Includes all of the filter options specified in the parameter array. -
not(PHPickerFilter)
: Includes all options except for the specific filter in the parameter.
Here is an example of a picker filter that will show only images.
let filter: PHPickerFilter = .images
Here is an example of a picker filter that will show images, panoramic photos and screenshots in the picker.
Only images in the PhotosPicker
UI.
let filter: PHPickerFilter = .all(of: [.images, .screenshots, .panoramas])
Here is an example of a picker filter that will show all asset types except videos.
let filter: PHPickerFilter = .not(.videos)
Here is an example of a picker filter that will show all asset except videos, slow-motion videos, bursts, Live Photos, screen recordings, cinematic videos and time-lapse videos.
let filter: PHPickerFilter = .not(.all(of: [
.videos,
.slomoVideos,
.bursts,
.livePhotos,
.screenRecordings,
.cinematicVideos,
.timelapseVideos
]))
As you can see, you can nest, mix and match filter options to suit your every need!
Adding Filtering to Photos Picker
How about adding some filters to only show images in the picker?
In MealDetail.swift, update the code that creates the PhotosPicker
as follows:
PhotosPicker(selection: $photosPickerItem, matching: .images) {
Label("Select Photo", systemImage: "photo")
}
For the matching
parameter, you specify images
as the only asset type that should show in the picker.
Build and run. Navigate to a meal’s details page. Open the photo picker.
You should only see images now. Whereas before, you would’ve seen all available asset types.
Polish & Wrap-Up
Depending on the device you’re using, loading and selecting an asset may or may not be immediate.
In this scenario, Apple recommends showing a non-blocking progress indicator, so your users know that you’re loading the asset after selection.
You may have noticed that there’s an isLoading
property already available inside of MealDetail
. Use it to show a ProgressView
inside your body’s HStack
, right after adding the PhotosPicker
:
if isLoading {
ProgressView()
.tint(.accentColor)
}
When isLoading
is true
, your users will see a ProgressView
next to the button.
Inside the onChange
modifier, update the Task
code block as follows:
Task {
isLoading = true
await updatePhotosPickerItem(with: selectedPhotosPickerItem)
isLoading = false
}
The code block helps determine whether the loading action is happening.
Build and run. Make a photo selection. You see something like this:
Things might go so fast that you don’t even get a glimpse of ProgressView
. It’s a good practice to add one anyway, as you shouldn’t assume users will use your app on the same device as you or under the same conditions.
If their experience is slower than the iOS simulator or a modern iOS device, then some indication of progress will go a long way toward avoiding frustration.
Fantastic work!
Where to Go From Here?
You can download the completed project files by clicking Download Materials at the top or bottom of the tutorial. Feel free to check out the final project to compare your results.
As you’ve just seen, using PhotosPicker
is easy and intuitive, and with very little code, you have a robust set of features available.
If you want to take the project further, how about adding the ability to add and edit meals as well as persisting them along with the selected photos? To learn how, check out the Saving Data in iOS course.
And check out the documentation for more on PhotosPicker
.
Hopefully, you found this tutorial as fun to read and follow as it was for me to write.
Thanks for your time, and feel free to leave any questions or comments in our forums.
Happy coding, and see you next time! :]