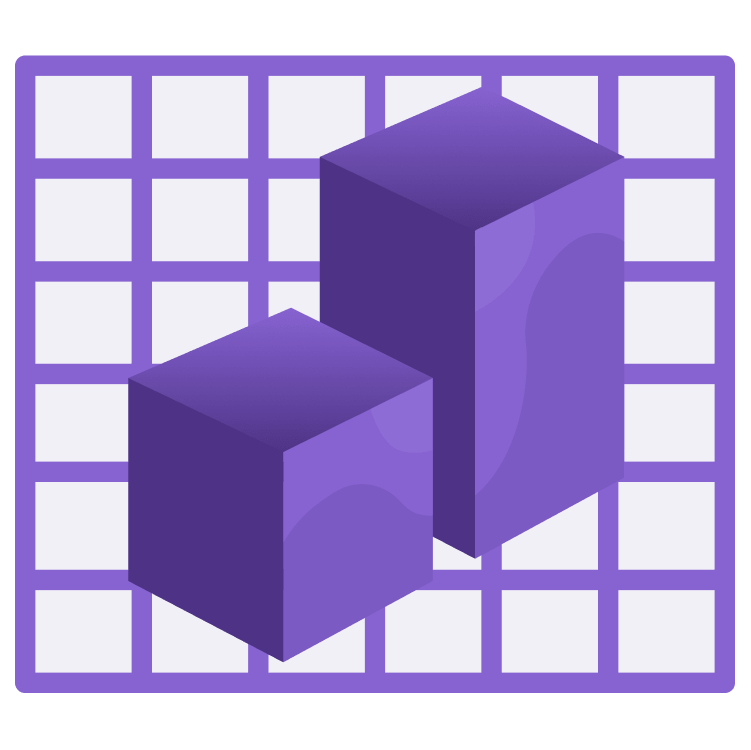
Swift Charts Tutorial: Getting Started
Learn how to use Swift Charts to transform data into elegant and accessible graphs. By Vidhur Voora.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Swift Charts Tutorial: Getting Started
35 mins
- Getting Started
- Getting Aquainted with Swift Charts
- Developing Charts
- Creating a Bar Chart
- Adding the Bar Chart
- Tidying up the Bar Chart
- Changing to a Horizontal Bar Chart
- Customizing the Bar Chart
- Using the Variants Feature in Xcode
- Fixing the Annotation
- Supporting Accessibility
- Putting it together
- Adding a Point Chart
- Customizing the Point Chart
- Adding a Line Chart
- Calculating and Creating the Line Chart
- Showing the Line Chart
- Customizing the Line Chart
- Finishing Up the Line Chart
- Combining Marks in a Line Chart
- Adding Drill-Down Functionality
- Adding Chart Type Picker
- Adding Multiple Marks
- Visualizing Multiple Data Points
- Where to Go From Here?
An attractive, well-designed chart is more useful to the user than rows and columns of data. If you need to make complex data simple and easy to understand in your app, this tutorial is for you!
Swift Charts is a flexible framework that allows you to create charts using the declarative syntax you’re already familiar with from SwiftUI. Out of the box, it supports dynamic font sizes, many screen sizes, and accessibility.
Before this framework existed, you had to create visualizations from scratch or use a third-party package.
Swift Charts gives you an elegant experience to create beautiful charts. You’ll add features to a starter app named WeatherChart. Your goal is to transform lists of historical weather data into appealing charts.
Along the way, you’ll:
- Learn about marks and properties — the building blocks for any Swift Chart.
- Create bar, line, area and point charts.
- Customize those charts.
- Improve the accessibility of the charts.
Are you ready to learn how to improve your apps with beautiful visualizations? Great! You can dive right in or use the navigation to jump ahead to a specific section.
Getting Started
Download the starter project by clicking the Download Materials button at the top or bottom of this page.
Open the WeatherChart project from the starter folder. You may remember this app from SwiftUI Tutorial for iOS: Creating Charts.
Build and run.
The app shows historical weather data from four stations in and around the Great Smoky Mountains National Park:
- Cherokee, NC and Gatlinburg, TN: The two cities on the main road through the park.
- Newfound Gap: The gap that intersects the main road.
- Mount LeConte: One of the highest mountains in the park.
The dataset contains each day’s precipitation, snowfall and temperature data.
Tap a location to show basic information about the location and a map of the area. Note the three tabs that show precipitation by month, daily snowfall and temperature ranges.
If you’re interested, you can review the raw data in weather-data.csv.
Getting Aquainted with Swift Charts
Take a moment to get familiar with the building blocks of any Swift chart: marks, properties, modifiers and data.
A mark is a graphical element that represents data; for example, the rectangular bars in a bar chart.
Swift charts include the following marks by default:
BarMark
PointMark
LineMark
AreaMark
RuleMark
RectangleMark
Marks are extensible, so you can create custom marks.
In this tutorial, you’ll use properties to provide data, and customize their appearance with modifiers.
Swift charts support three types of data:
- Quantitative: represents numerical values, such as temperature, inches of snowfall, etc.
- Nominal: values are discrete categories or groups, such as a city, name of a person, etc. This data type often becomes the labels.
- Temporal: represents a point or interval in time, such as the duration of a particular day part.
There’s more to learn, but this is enough to get you started and into the next part, where you actually get to build something.
Developing Charts
Enough theory — it’s time to start the hands-on part of this tutorial. From here to the end, you’ll develop and change several charts.
By the time you reach the end of this tutorial, you’ll have hands-on experience creating marks and modifying their properties.
Creating a Bar Chart
Your first task is to create a bar chart for the precipitation data. A bar chart provides a bar for each data point. The length of each bar represents a numerical value, and it can be horizontally or vertically oriented.
Go to the Tabs group and open PrecipitationTab.swift.
You’ll see a standard SwiftUI List()
that loops through the integers 0 through 11, representing the months of the year. It displays the total precipitation in inches for each month.
Expand the Charts group and open PrecipitationChart.swift. This is currently an empty view. Add the following variable to PrecipitationChart
:
var measurements: [DayInfo]
With this, you pass the weather data to measurements from PrecipitationTab.
Replace the content of previews
in PrecipitationChart_Previews
with:
// swiftlint:disable force_unwrapping
PrecipitationChart(
measurements: WeatherInformation()!.stations[2].measurements)
Here you pass weather data in for the preview.
Next, add a helper method to PrecipitationChart
:
func sumPrecipitation(_ month: Int) -> Double {
self.measurements.filter {
Calendar.current.component(.month, from: $0.date) == month + 1
}
.reduce(0) { $0 + $1.precipitation }
}
This short block of code does a lot:
-
sumPrecipitation(_:)
takes anInt
to represent the month. -
filter
gets the measurements for that specific month then adjusts for the integer which is passed in as a zero index — this adjusts it to one. -
reduce
totals the precipitation values for those measurements.
Next, add the following below import SwiftUI
:
import Charts
Here, you import the Charts
framework.
Adding the Bar Chart
Replace the contents of body
with:
// 1
Chart {
// 2
ForEach(0..<12, id: \.self) { month in
// 3
let precipitationValue = sumPrecipitation(month)
let monthName = DateUtils.monthAbbreviationFromInt(month)
// 4
BarMark(
// 5
x: .value("Month", monthName),
// 6
y: .value("Precipitation", precipitationValue)
)
}
}
Here’s what is going on in there:
- Start creating a chart by adding a
Chart
struct. Then declare marks and set the corresponding properties within its body. - Add a
ForEach
loop to generate a bar chart for each month. - Use two utility methods to:
- Get the sum of the precipitation data for the month.
- Get the abbreviated month name by passing the month number to
monthAbbreviationFromInt(_:)
fromDateUtils
.
- Create a
BarMark
for the chart to show the bars — marks denote the visual elements. - Set the name of the month to the
x
argument. The first argument to.value
modifier is the description of the value. The second argument is the actual value itself. - Set the sum of the monthly precipitation data as the value — the height of each bar is controlled by
y
argument.
- Get the sum of the precipitation data for the month.
- Get the abbreviated month name by passing the month number to
monthAbbreviationFromInt(_:)
fromDateUtils
.
Turn your attention to the preview window. The bar chart should now show precipitation data for each month.
Notice how Swift Charts elegantly used the abbreviated month name as a label for each bar along the x-axis. The y-axis is also set to an appropriate range based on the provided rainfall data.
Pretty cool! Pat yourself on the back and treat yourself to a candy bar for raising the bar...with a bar! :]