Working With Arrays Within Objects
The Gifts department wants to see both the names and the labels of the employees’ birthday gifts, so its API generates JSON that looks like this:
{
"name" : "Teddy Bear",
"label" : [
"teddy bear",
"TEDDY BEAR",
"Teddy Bear"
]
}
You nest the label names inside label
. The JSON structure adds an extra level of indentation compared to the previous challenge, so you need to use nested unkeyed containers for label
in this case.
Open Nested unkeyed containers and add the following code to Toy
:
func encode(to encoder: Encoder) throws {
var container = encoder.container(keyedBy: CodingKeys.self)
try container.encode(name, forKey: .name)
var labelContainer = container.nestedUnkeyedContainer(forKey: .label)
try labelContainer.encode(name.lowercased())
try labelContainer.encode(name.uppercased())
try labelContainer.encode(name)
}
Here you are creating a nested unkeyed container and filling it with the three label values. Run the playground and inspect string
to check the structure is right.
You can use more nested containers if your JSON has more indentation levels. Add the decoding code to the playground page:
extension Toy: Decodable {
init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
name = try container.decode(String.self, forKey: .name)
var labelContainer = try container.nestedUnkeyedContainer(forKey: .label)
var labelName = ""
while !labelContainer.isAtEnd {
labelName = try labelContainer.decode(String.self)
}
label = labelName
}
}
let sameToy = try decoder.decode(Toy.self, from: data)
This follows the same pattern as before, working through the array and using the final value to set the value of label
, but from a nested unkeyed container.
Congratulations on completing all the challenges! :]
Encoding and decoding in Swift like a pro!
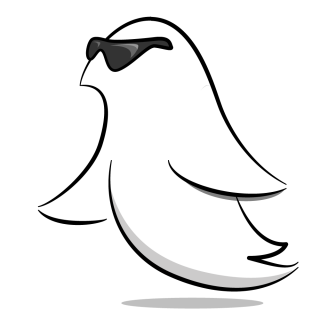
Encoding and decoding in Swift like a pro!
Where to Go From Here?
Download the final playground using the Download Materials button at the top or bottom of the tutorial.
If you want to learn more about encoding and decoding in Swift, check out our Saving Data in iOS video course. It covers JSON
, Property Lists
, XML
and much more!
I hope you enjoyed this tutorial and if you have any questions or comments, please join the forum discussion below! :]