It’s ARRAYning Quotes
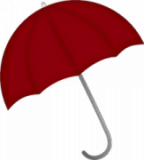
Just for fun, you’re going to concatenate all your quotes together, as there aren’t many. This will illustrate how to loop through an array, which you might need to do if you want to iterate over each item in an array to process each item.
In quoteButtonTapped: replace section #1 with the following:
if (self.quoteOpt.selectedSegmentIndex == 2) {
// 1.1 - Get array count
int array_tot = [self.myQuotes count];
// 1.2 - Initialize string for concatenated quotes
NSString *all_my_quotes = @"";
NSString *my_quote = nil;
// 1.3 - Iterate through array
for (int x=0; x < array_tot; x++) {
my_quote = self.myQuotes[x];
all_my_quotes = [NSString stringWithFormat:@"%@\n%@\n", all_my_quotes,my_quote];
}
self.quoteText.text = [NSString stringWithFormat:@"%@", all_my_quotes];
}
A for loop is used to loop through the array from row 0 to the last row. x is the counter that is used to keep track of the rows.
Now run and check the results.
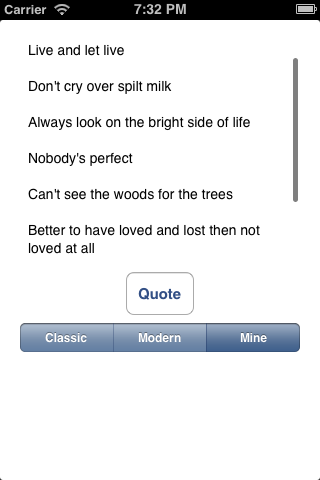
One last thing. I mentioned earlier that there are different types of arrays: NSArray and NSMutableArray. So far, each has done the same job in this project.
Use an NSMutableArray if you want to modify/update it. As the name implies, a mutable array can be changed whereas a normal NSArray is static and you cannot add new items to the array or delete items from the array easily.
For example, if you wanted to update the array after a row had been selected in order to show that that quote had already been displayed, you would need an NSMutableArray.
In this project, movieQuotes is your NSMutableArray. You're using a predicate, so you first need to find the row in movieQuotes and then update it. Add the following code after section #2.9 in quoteButtonTapped::
// 2.10 - Update row to indicate that it has been displayed
int movie_array_tot = [self.movieQuotes count];
NSString *quote1 = filteredArray[index][@"quote"];
for (int x=0; x < movie_array_tot; x++) {
NSString *quote2 = self.movieQuotes[x][@"quote"];
if ([quote1 isEqualToString:quote2]) {
NSMutableDictionary *itemAtIndex = (NSMutableDictionary *)self.movieQuotes[x];
itemAtIndex[@"source"] = @"DONE";
}
}
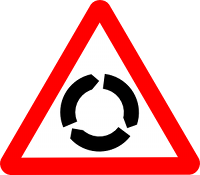
You loop through the array and check each row to see if it's the row you're looking for. Again you use isEqualToString; this time, however, you're comparing two string variables.
To update the row in the array, you retrieve the object for the row in question and update the object. This is starting to get a bit advanced for this tutorial, but it's useful to know.
Since you're updating the source string and since the source string is what is used to select quotes for each category, the row will not be included next time you use NSPredicate to filter the array. And that's quite neat.
Where to Go From Here?
Here is a sample project with all of the code from the above tutorial.
Well, you've reached the end of this little project. You've learned how to use arrays in different ways, initiate actions from interface events, access objects via Interface Builder using a XIB file and do various string comparisons and concatenations. That is not a bad effort if this is your first iPhone app!
Now that you know the basics, you might want to try out our How To Create a Simple iPhone App tutorial series, or sign up for our monthly newsletter for an epic-length iOS tutorial for beginners.
The forums are available if you have any questions about what you've done. Also, if you liked this tutorial and would like to see more in the series, please let me know in the forums!
In the meantime, good luck and keep 'appy! :]