Saving View State
You can save your view state in case there is any change in the device configuration, e.g., orientation, by overriding the onSaveInstanceState()
and onRestoreInstanceState()
methods.
Add the following method overrides to EmotionalFaceView
:
override fun onSaveInstanceState(): Parcelable {
// 1
val bundle = Bundle()
// 2
bundle.putLong("happinessState", happinessState)
// 3
bundle.putParcelable("superState", super.onSaveInstanceState())
return bundle
}
override fun onRestoreInstanceState(state: Parcelable) {
// 4
var viewState = state
if (viewState is Bundle) {
// 5
happinessState = viewState.getLong("happinessState", HAPPY)
// 6
viewState = viewState.getParcelable("superState")
}
super.onRestoreInstanceState(viewState)
}
Here you:
- Create anew
Bundle
object to put your data into.
- Put the happiness
state
value into the bundle.
- Put the state coming from the superclass, in order to not lose any data saved by the superclass, then return the
bundle
.
- Check the type of the
Parcelable
to cast it to a Bundle
object.
- Get the
happinessState
value.
- Get the superstate then pass it to the super method.
Build and run the app, change the happiness state to sad, and change the orientation of your device. The center face should remain sad after device rotation:
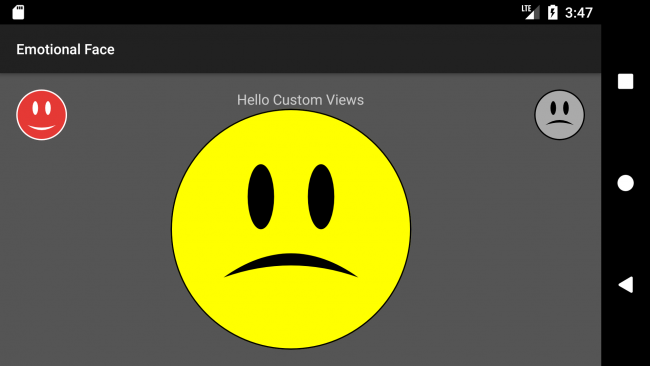
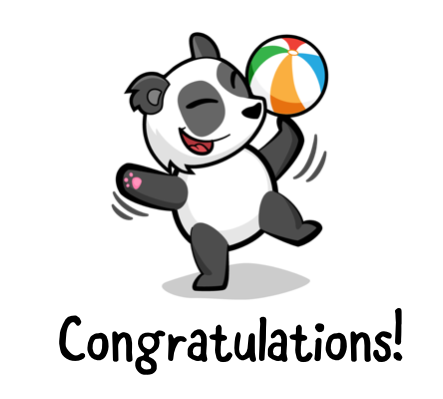
Where To Go From Here?
Yes! You have created your own custom view :]
You can download the completed project using the download button at the top or bottom of this tutorial.
During this tutorial you:
- Drew a circle, oval and a path on a canvas. You can learn more about custom drawing here.
- Made the custom view responsive.
- Created new XML attributes for the custom view.
- Saved the view state.
- Reused the custom view for different use cases.
You can make your custom view even more interactive by detecting special gestures; check for more info here.
Adding animation to your custom view can enhance the UX strongly. Check out Android Animation Tutorial with Kotlin.
You can also learn more about drawable animation and Canvas and Drawables.
Feel free to share your feedback or ask any questions in the comments below or in the forums. Thanks!