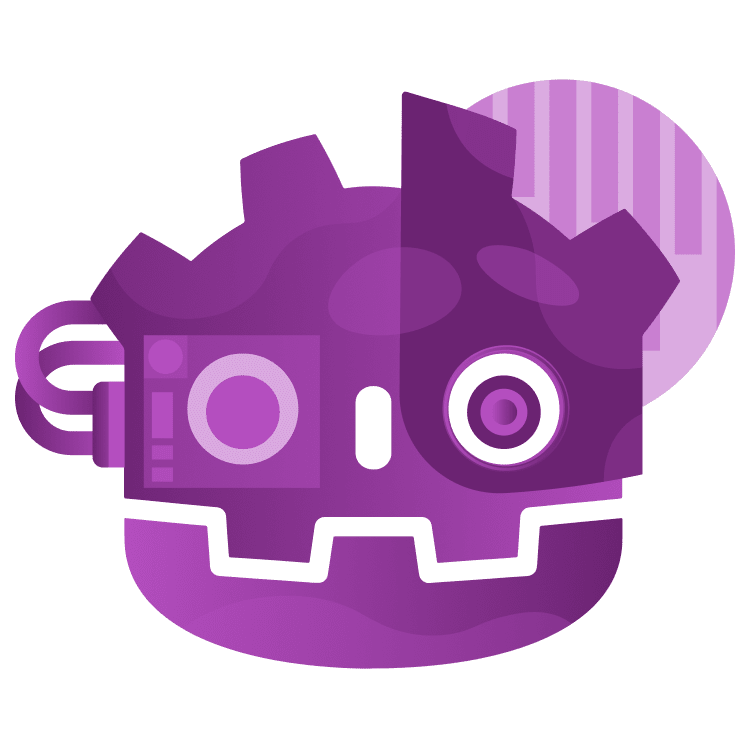
Extending the Editor with Plugins in Godot
Embark on a journey to harness the true power of Godot with editor plugins! Revolutionize your workflow with bespoke tools, efficient shortcuts, and personalized menu options. Delve deep into the art of plugin creation and unlock the boundless potential of Godot with ease. By Eric Van de Kerckhove.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Extending the Editor with Plugins in Godot
45 mins
- Getting Started
- Plugin Overview
- Creating Your First Plugin
- Scaffolding
- Taking a Closer Look
- Handling Node Selection
- Adding the Button
- Developing an Advanced Plugin
- Creating the Menu Scene
- Loading and Unloading the Menu
- Getting Selected Nodes
- 2D Physics in Godot
- Starting and Stopping Physics
- Custom Physics Spaces
- Physics Frames
- Adding Quality of Life Features
- Where to Go From Here?
Plugins are a great way to add functionality to Godot’s editor with minimal effort. They allow you to add features like custom tools, useful shortcuts, and menu items to speed up your workflow. This article will guide you through the process of creating your own plugins to unlock the full potential of Godot.
Getting Started
To get started, download the project materials via the Download Materials link at the top and bottom of this page. Next, open the EditorPlugins project you find in the starter folder and open it in Godot.
Before delving into plugins, I’ll give a quick tour of the project. It comes with a single scene named main.tscn and some sprites. Open the main scene and take a look at the nodes inside.
There’s a collection of StaticBody2D and RigidBody2D nodes here that make up a non-interactive physics scene. If you direct your attention to the Scene dock, you’ll see there are all named according to their function.
Notice that only the top level nodes can be selected and moved, while their children are locked. This is intentional to avoid moving the collision shapes and sprites by themselves by accident.
Now try running the project by pressing F5 and see what happens.
Shortly after running the project, you’ll see the little blue avatar falling down on a red box, while the other red box falls off a platform. There’s no actual gameplay involved here as the focus is on extending the editor.
Now you know your way around the scene, it’s time to learn about plugins!
Plugin Overview
In Godot, plugins are scripts that extend the functionality of the editor. You can write these scripts using GDScript or C# and there’s no need to recompile the engine to make them work. Besides the main plugin script, you can add scenes and extra scripts to the plugin to make it even more versatile. These work the same way like the rest of your project, meaning you can use your existing knowledge of Godot to extend it!
You can use plugins to add buttons, shortcuts and even whole new screens to the editor. Here are some examples of what you can do with plugins:
- Create a conversation manager
- Integrate Google Sheets into your project to load data from
- Add custom resource importers
- Make your own node types
- Automatically create the right size of colliders for sprites
These are just a handful of ideas to give you a taste of what you can do with plugins. If you ever thought about a feature you’d want in Godot, there’s a good chance you can find it in a plugin or create your own.
Creating Your First Plugin
For your first plugin, you’ll add a button to the toolbar that toggles the visibility of a selected node.
Scaffolding
Plugins need three things to work:
Thankfully, Godot makes it easy to create new plugins as it’s built into the editor. To get started, open the Project Settings via Project ▸ Project Settings… in the top menu. Now select the Plugins tab and you should see an empty list.
- They need to be in a folder named addons in the root of the project
- They need a file named plugin.cfg containing the metadata
- They need at least one script that derives from
EditorPlugin
Thankfully, Godot makes it easy to create new plugins as it’s built into the editor. To get started, open the Project Settings via Project ▸ Project Settings… in the top menu. Now select the Plugins tab and you should see an empty list.
Now click the Create New Plugin button and the Create a Plugin dialog window will pop up.
Godot will create the necessary files for you based on the information you provide, pretty neat! For this visibility button plugin, fill in the following information:
- Plugin Name: Visibility Button
- Subfolder: visibility_button
- Description: Easily show and hide a selected node.
- Author: Your name or username
- Script Name: visibility_button.gd
Here’s what it should look like:
Next, click the Create button to let Godot create the necessary files for you. This will also automatically open the visibility_button.gd script in the script editor.
Before editing the script, take a look at the files and folders Godot has created for you in the FileSystem dock.
There’s a new folder named addons now that contains a folder for the plugin named visibility_button. Inside that folder, there’s a file named plugin.cfg and a script named visibility_button.gd. plugin.cfg contains the metadata for the plugin, while the script contains the actual code.
EditorPlugin
.
Enough scaffolding, time to take a look at the code!
Taking a Closer Look
The visibility_button.gd script that Godot generated for you contains the following code:
@tool # 1
extends EditorPlugin # 2
func _enter_tree() -> void: # 3
# Initialization of the plugin goes here.
pass
func _exit_tree() -> void: # 4
# Clean-up of the plugin goes here.
pass
I took the liberty to add some numbered comments to make it easier to explain what each line does:
- The
@tool
annotation turns a regular script into a tool script. This means that any code in the script will be executed in the editor. This is powerful, but it also makes it easy to break entire scenes when not careful. You’ll learn more about the@tool
annotation in another article. If you want to know more about it in the meantime, check out the Running code in the editor page of Godot’s documentation. - All editor plugins must inherit from
EditorPlugin
. This class comes with a ton of useful functions to access and edit Godot’s editor. - The
_enter_tree
function is called when you activate the plugin. This where you set up all needed variables and references for later use. - The
_exit_tree
function is called when you disable the plugin. This is where you clean up all references.