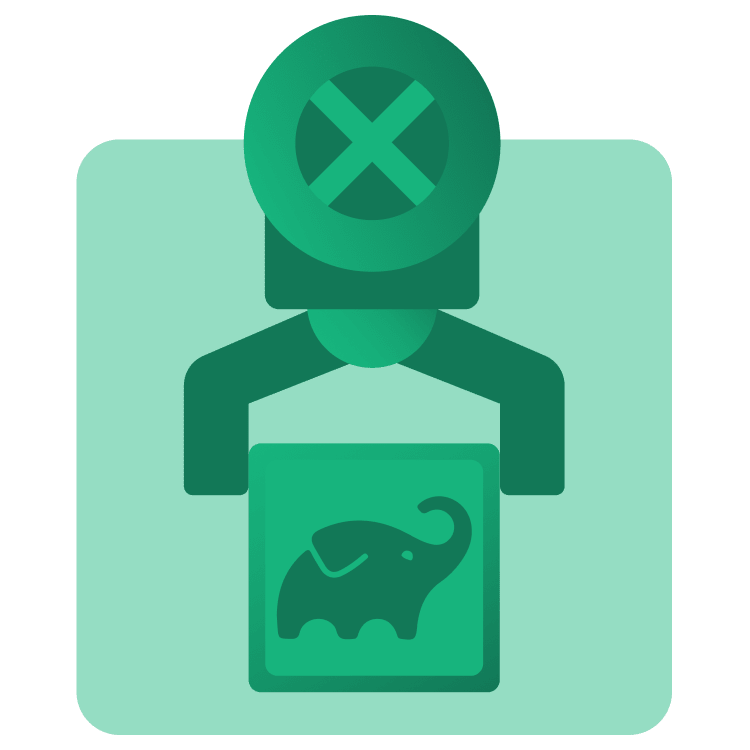
Gradle Tutorial for Android: Getting Started – Part 1
In this Gradle Build Script tutorial, you’ll learn the basic syntax in build.gradle files generated by Android Studio. You’ll also learn about gradlew tasks, different dependency management techniques, and how to add a new dependency to your app. By Ricardo Costeira.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Gradle Tutorial for Android: Getting Started – Part 1
35 mins
- What is Gradle?
- Getting Started
- Exploring the Project-Level Files
- Moving on to Module-level Files
- Graduating to the Settings Files
- Using New(er) Gradle Configurations
- Mastering the Build: Gradle Commands
- Defining gradlew
- Executing gradlew Tasks
- gradlew assemble
- gradlew lint
- Managing Dependencies
- Using the ext Property
- Using buildSrc
- Using Version Catalogs
- Including Picasso
- Configuring Gradle Dependency
- Where to Go From Here?
Including Picasso
Oof, that was a lot of work. You’ll now do some work on the app itself. But before, and using the dependency management system of your preference, include Picasso in the module-level’s build.gradle.kts or build.gradle file — depending if you’re using Kotlin or Groovy —, under the dependencies
block:
- If using
ext
, addimplementation "com.squareup.picasso:picasso:$picasso"
. - With
buildSrc
, addimplementation(Dependencies.picasso)
. - If you prefer Version Catalogs, add
implementation(libs.picasso)
for Kotlin, orimplementation libs.picasso
for Groovy.
Sync the project. When ready, open ProfileActivity
and add this function to load a user’s avatar:
// 1
private fun loadAvatar() {
// 2
Picasso.get().load("https://goo.gl/tWQB1a").into(binding.avatar)
}
Also, be sure the following import is included:
import com.squareup.picasso.Picasso
Going step-by-step:
- You define a Kotlin function
loadAvatar()
- You get a Picasso instance through the `get()` method. Next, you specify the URL of an image you want to load with the
load(path: String!)
method. The only thing left is to tell Picasso where you want this image to be shown by calling theinto(target: ImageView!)
method.
Add this function invocation at the end of onCreate(savedInstanceState: Bundle?)
method of your ProfileActivity
:
loadAvatar()
The last step, which even senior Android developers tend to forget, is to add the android.permission.INTERNET
permission so that Picasso can download the image. Go to the AndroidManifest.xml file and add the following permission above the application
tag:
<uses-permission android:name="android.permission.INTERNET" />
That’s all you need to show the user’s avatar. Build and run the project:
Configuring Gradle Dependency
The implementation
keyword you previously used is a dependency configuration, which tells Gradle to add Picasso in such way that it’s not available to other modules. This option significantly speeds up the build time. You’ll use this keyword more often than others.
In some other cases, you may want your dependency to be accessible to other modules of your project. In those cases, you can use the api
keyword.
As an example, let’s say you have a library module on your app, called ui
. In that module, you include Picasso like so:
dependencies {
// ...
api(libs.picasso)
}
Then, in your app
module, you include the ui
module:
dependencies {
// ...
implementation(project(":ui"))
}
By including Picasso in the ui
module through api
, you make it accessible to the app
module as well, even if you don’t explicitly include it in this module. This is not something you want to abuse though, as any dependency added to a module through api
becomes part of that module’s public API — see where the keyword comes from?
In other words, changing a dependency added through api
will force a recompilation of any modules that depend on the module the dependency is included in, just like it will happen if you change one of the public interfaces/methods in that module. That said, it can be useful in some cases — to enforce specific dependencies, for instance — but use it sparingly.
Apart from api
and implementation
, there other options like runtimeOnly
and compileOnly
configurations, which mark a dependency’s availability during runtime or compile time only.
Where to Go From Here?
Download the completed project files by clicking the Download Materials link at the top or bottom of the tutorial.
If you want to know more about Android’s build system, continue on to official documentation.
To learn about more advanced Gradle concepts like plugins and tasks, you can check the part 2 of this tutorial.
We hope you enjoyed this tutorial. If you have any questions or comments, please join the forum discussion below!