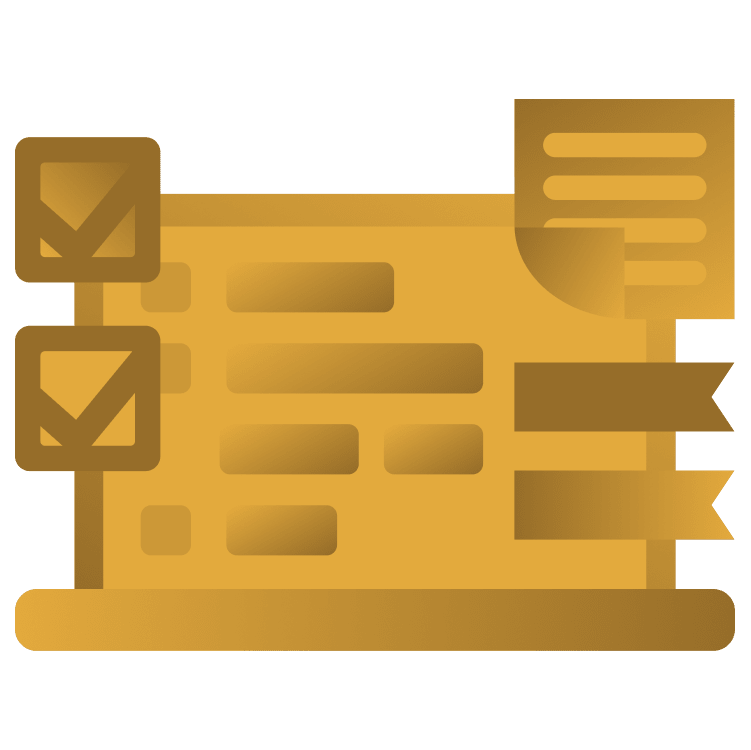
Code Comments: How to Write Clean and Maintainable Code
Discover the benefits of good code comments and learn best practices for writing effective documentation. Improve code quality, readability, and collaboration. By Roberto Machorro.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Code Comments: How to Write Clean and Maintainable Code
20 mins
- What Are Code Comments?
- A Brief History of the Comment
- Code Without Comments
- Good Comments
- Useless Comments
- Bad Comments
- Beyond Annotations
- LICENSE Comments
- TEMPORARY CODE-OUT Comments
- DECORATIVE Comments
- LOGIC Comments
- Advanced Forms of Comments
- Flag Comments
- TASK Comments
- DOCUMENT GENERATION Comments
- CDATA Comments
- Having Fun With Comments
- Key Takeaways
- Where to Go From Here?
- About the Author
If you spend a reasonable amount of time programming, you’re sure to encounter code comments. You may have even written some yourself. These tiny pieces of text are embedded within the program, but they’re ignored by the compiler or interpreter. They’re intended for the humans who work with the code, and they have many applications. They may even reshape your code or create documentation for others automatically. Want to know how to use them properly? Then you’re in the right place!
Time to explore comments, how to use them and also how not to use them.
What You’ll Learn:
By reading this article, you’ll learn:
- What a comment is, types and how to use them.
- Where they began and where they are now.
- The difference between good and bad comments.
- Practical applications from the past and present.
By the end of the article, you’ll know why code comments are important, best practices for writing effective comments, some common pitfalls and mistakes to avoid and the tools and resources you can use to optimize your code commenting process, including automated documentation generators, comment linters and code review platforms.
You’ll start with an in-depth look at what code comments are.
What Are Code Comments?
Comments within a software program are human-readable annotations that programmers add to provide information about what the code is doing. Comments take many forms, depending on the programming language and the intent of the comment.
They’re categorized into two types: block comments and line comments.
-
Block comments delimit a region within the code by using a sequence of characters at the start of the comment and another character sequence at the end. For example, some use
/*
at the start and*/
at the end. -
Line comments use a sequence of characters to ask the compiler to ignore everything from the start of the comment until the end of the line. For example:
#
,–
or//
. How to indicate line comments depends on the programming language you’re using.
Here’s an example of how each kind of comment looks in Swift:
struct EventList: View {
// THIS IS A LINE COMMENT @EnvironmentObject var userData: UserData
@EnvironmentObject var eventData: EventData
/* THIS IS A BLOCK COMMENT
@State private var isAddingNewEvent = false
@State private var newEvent = Event()
*/
var body: some View {
...
}
}
Some programming languages only support one type of comment sequence, and some require workarounds to make a sequence work. Here’s how some languages handle comments:
Think about how you’d use a sticky note. For programmers, a comment is a sticky note with unlimited uses.
Comments have taken on more importance over time as their uses have expanded and standardized. You’ll learn about many of these uses in this article.
A Brief History of the Comment
Not so long ago, computers weren’t programmed using a screen and a keyboard. They were programmed using punch cards: small pieces of stiff paper with holes punched in a sequence to indicate instructions for a computer. These would become giant stacks that were fed into a reader, and the computer would run the program.
It was hard to know at a glance what each card or a set of cards did. To solve this, programmers would write notes on them. These notes were ignored by the machine reader because it only read the holes on the card. Here’s an example:
Today, most comments serve the same purpose: to assist in understanding what code does.
Code Without Comments
A common saying among programmers is, “Good code doesn’t need an explanation”. This is true to a certain extent, and some programmers don’t write comments in their code.
But what happens when that code gets really big? Or it incorporates several business rules and logic? Or other people need to use it? It would be possible to decipher the uncommented code, but at what cost in time and effort?
When our focus is constantly on writing code, we forget that a few months — even a few weeks — after writing said code, a lot of the logic has left our memory. Why did we make certain choices? Why did we use certain conventions. Without comments, we will have to go through the expensive exercise of figuring out what we were thinking.
Comments are incredibly useful, and good developers should use them. However, all comments are not created equal. Here’s a look at what makes comments good, useless or even counterproductive.
Good Comments
Good comments are simple and easy to read. They clarify or indicate a train of thought as opposed to how the code works.
They take many forms, such as annotations, links and citations to sources, licenses, flags, tasks, commands, documentation, etc.
Useless Comments
Comments are good, but you don’t need to use them everywhere. A common pitfall is using comments to describe what code is doing, when that’s already clear.
For example, if you have code that’s iterating through an array and incrementing each value, there’s no need to explain that — it should be obvious to the programmer who’s reviewing the code. What isn’t obvious, though, is why you’re doing that. And that’s what a good comment will explain.
Describing the obvious is a common pitfall… and comments that do this are just taking up space and potentially causing confusion.
Bad Comments
Bad comments are more destructive than useless. They cause confusion, or worse, try to excuse convoluted or badly written code. Don’t try to use comments as a substitute for good code. For example:
var n = 0 // Line number
Instead, do:
var lineNumber = 0
Abusing comments, such as cluttering code with TODO, BUG and FIXME comments, is bad practice. The best solution is to resolve these prior to committing code when working on a team — or simply create an issue in a ticketing system.
Since comments are free-form, it’s helpful to have some conventions or templates to determine what a comment should look like, but mixing conventions falls into the category of bad comments.
Did you comment on code during development? Don’t commit it — resolve the problem and take out the comment before you confuse others in the team.