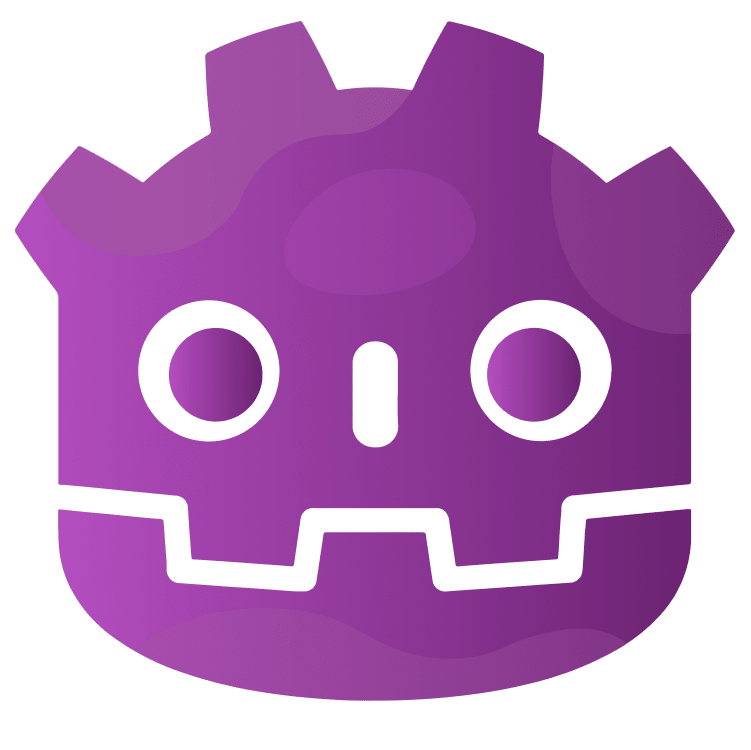
Godot 4: Getting Started
Learn the basics of how to use Godot 4 to create games and applications in this tutorial aimed at beginners. By Eric Van de Kerckhove.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Scripting
Scripting is a fundamental skill when working with any game engine, including Godot. A script is a set of commands that tell the engine what to do and can extend its functionality. In Godot, scripts can be attached to nodes to add new features and behaviors to them.
For example, a Sprite2D node by itself can only display a texture, but with a script, it can follow the cursor, jump, or fade away. Scripts like these can be used to call web APIs, calculate formulas, and perform other actions necessary for creating a game or application.
Godot supports two main programming languages for scripts: GDScript and C#. Additionally, Godot allows for the use of GDExtension, a high-performance method for expanding the engine with libraries written in C or C++. This tutorial will focus solely on GDScript, as it’s an object-oriented language specifically designed for Godot. Its syntax is similar to Python and is widely used in Godot documentation and tutorials.
Your First Script
It’s tradition to start every introduction to a programming language with a “Hello World”, but for this example the nodes will tell you their name instead to greet you into the world of Godot.
To start with, open the icon scene again by clicking its tab at the top or by clicking its scene file in the FileSystem dock. Now select the Icon node via the Scene dock and click the script button at the top right of the Scene dock, it looks like a scroll with a green plus.
This opens the Attach Node Script window.
By default, a new GDScript will be created that builds upon the selected node type, Sprite2D. The path where the script will be places should be changed, so click the folder button next to the Path property. In the window that opens, go up one directory with the up arrow at the top left and create a new folder named “scripts” in the project root directory.
Now click the Open button at the bottom to choose the scripts folder as the location for your new script. Back in the Attach Node Script window, click the Create button at the bottom to create a new script named icon.gd. This will open up the script in Godot’s built-in code editor automatically:
extends Sprite2D
# Called when the node enters the scene tree for the first time.
func _ready():
pass # Replace with function body.
# Called every frame. 'delta' is the elapsed time since the previous frame.
func _process(delta):
pass
Without delving too deep into scripting, here’s what you should know about this code:
- The
extends Sprite2D
part at the top makes it so this script can access all Sprite2D node properties, like its texture and transform. -
func _ready()
is a function that gets called by the engine once at the start of the node’s lifetime. - The
_process
function gets called every frame by the engine. - The
pass
keyword doesn’t do anything here except for exiting the functions. They act as placeholders for your own code.
GDScript uses tabs to group functions and statements together. These show up in the code editor as >|
.
This is important to note as you might get an error saying something similar to “Expected indented block after function declaration.” if you forgot to add a tab or remove one by accident.
With that small disclaimer out of the way, it’s time to finally add your own code. Replace the pass
in the _ready
function with this print
call:
print("Hello, I'm ", name)
The print
method takes one or more arguments and prints them to the output console. The name
variable is part of every node; it’s the name that you gave it via the Scene dock earlier: Icon.
Now save the script by pressing CTRL/CMD+S and run the icon scene by pressing F6. Next, minimize or drag away the the game window so you can see Godot’s editor and take a look at the output window at the bottom. There should be a new text entry in there saying “Hello, I’m Icon”.
Great work! You just added and ran your first script in Godot. Press the Stop Running Project button at the top right to close the game window
To add something to look at in the main scene, you’re going to make the icons rotate. To do that, remove the pass
keyword from the _process
function and replace it with the following:
rotate(delta)
The rotate
method is part of Node2D (the parent node class of Sprite2D) and applies rotation to a node. The delta
variable is passed to the _process
function by the engine, it’s the time in seconds between the previous and current frame. By using a delta time as the rotation value instead of a constant value like 5 for example, the rotation will be smooth and framerate-independent.
In the games of yore, the speed of a game was tied to the CPU speed, which meant that some games are hardly playable because everything whizzes by so fast. Meanwhile, that same game on a slow CPU would result in everything moving in slow-motion. Using the delta time ensures a reliable, constant change in value based on time instead of processor speed. Don’t worry if this concept is still vague to you at this point, just remember to use the delta when you need something to constantly change over time.
Now save the script using the now familiar CTRL/CMD+S shortcut and press F5 to run the main scene, game. If all went well, you’ll see the icons you added earlier rotating.
Meanwhile, if you take a look at the output window in the editor, you’ll see that the icons are telling you their name.
That concludes this tutorial! I hope you had fun while taking your first steps with Godot as I’m looking forward to writing more about it in the future.