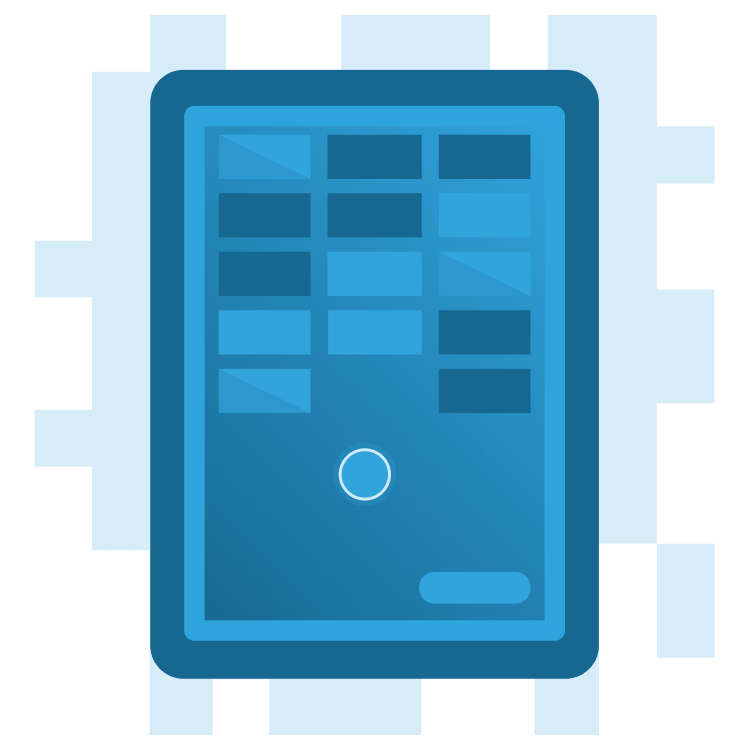
Create a Breakout Game in Flutter With Flame and Forge2D – Part 1
Learn how to create a Flutter version of the classic Breakout game using Flame and Forge2D. By Michael Jordan.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Create a Breakout Game in Flutter With Flame and Forge2D – Part 1
30 mins
- Getting Started
- Breakout Game Requirements
- Understanding the Flame Game Engine and Forge2D
- Adding Flame and Forge2D Dependencies
- Setting up Your Flame Game Loop
- Creating the Ball
- Defining a Ball’s Physical Properties
- Creating a Game Arena
- Understanding Forge2D Units and Coordinates
- Units
- Coordinate Systems
- The Flame Camera
- Breakout Game Metrics
- Adjusting the Camera, Arena and Ball Body Properties
- Where to Go From Here?
As Flutter continues to mature and expand its capabilities on multiple platforms, it’s also branching out to embrace new software domains like game development. As a result, more indie developers are jumping on the bandwagon and creating great games using Flutter.
You have several options for building a game in Flutter. Your choice will largely depend on the type of game you want to create. For example, Filip Hráček created a tic-tac-toe game using just Flutter widgets. Vincenzo Guzzi built a 2D orthographic game in his excellent article Building Games in Flutter with Flame: Getting Started using Flame.
This is the first of three articles that show you how to build a version of the classic game Breakout using Flame and Forge2D, a two-dimensional physics simulator engine for games.
Here’s what you’ll learn in each part:
- In Part 1, you’ll learn the basics of creating a Forge2D game using Flutter and Flame. Then, you’ll learn how to set up the game loop and create rigid bodies in a simulated two-dimensional physical world. By the end of the article, you’ll have created a Forge2D game with a ball that ricochets off the walls of a contained area.
- In Part 2, you’ll continue building the remaining components for your Breakout game. You’ll learn how to build a brick wall and a user-controlled paddle. By the end of the article, you’ll have all the essential elements for your Breakout game.
- And finally, in Part 3, you’ll learn how to add gameplay logic and skin your game with the visual, completing the look and feel for a playable Breakout game.
Getting Started
Building a Breakout game in Forge2D is a big enough challenge that it makes sense to tackle the task in three parts. In the first part of your journey, you’ll learn how to:
- Create a Flame
GameWidget
with aForge2DGame
child widget. - Create Bodies and Fixtures, the component building blocks of a Forge2D world.
- Work with Forge2D world coordinates and learn how they relate to Flutter’s logical pixels.
- Learn about the Flame
Camera
and viewing into the Forge2D world.
You’ll need the starter project to complete this tutorial. Download it by clicking the Download Materials button at the top or bottom of the tutorial.
Open the project in your preferred IDE. This tutorial used Visual Studio Code, but any Flutter development environment should work. Next, open pubspec.yaml and get the project dependencies, then build and run the project.
You’ll see a green border and the text Flame Game World Goes Here! centered on the display.
The screen images in this tutorial are from the iOS Simulator, but the app will run and look similar on Android or a Chrome browser.
Take a moment to familiarize yourself with the starter project. This project is a minimal Flutter app with a simple lib/main.dart implementation that creates an instance of the MainGamePage
widget. Look at the MainGameState
widget class in lib/ui/main_game_page.dart, and you’ll see a Scaffold
widget with a Container
widget for the body. In this tutorial, you’ll replace the Container
‘s child widget, the Center
widget, with a Flame GameWidget
. The GameWidget
will contain the Forge2D world of your Breakout game.
Breakout Game Requirements
The game’s objective is simple — destroy all the bricks in the wall by repeatedly bouncing the ball off a paddle. Each time the ball hits a brick, that brick is destroyed. Eliminate all bricks, and you win the game. Miss the ball, and you lose the game.
Breakout consists of three components:
- A ball in motion.
- A user-controlled paddle.
- A wall of bricks.
To create the game, you need to draw a ball on-screen and update its position in a way that simulates the motion of a ball in the real world. Then, you’ll need to detect when the ball comes into contact with a brick, the paddle or the sides of the game area, then have the ball bounce off them as a ball would in the real world. Players expect the ball’s behavior in Breakout to mimic real-world examples like tennis or handball. Otherwise, its behavior would be confusing or unexpected to the player.
While you can create a Breakout game using Dart and Flame alone, you would have to perform all the calculations for the physical interactions between the ball, the paddle and the bricks. That’s a lot of work! Here’s where Forge2D comes to the rescue.
Understanding the Flame Game Engine and Forge2D
Forge2D is a two-dimensional physics simulator specifically designed for games. Forge2D integrates with the Flame game engine to work with Flame’s game loop to update and render objects while obeying Newton’s three laws of motion. So, you can create the ball, paddle and a wall of bricks in Forge2D and then let it do all the heavy lifting.
Adding Flame and Forge2D Dependencies
Begin by opening the pubspec.yaml file in your project, and add the flame and flame_forge2D packages:
dependencies:
flame: ^1.4.0
flame_forge2d: ^0.12.3
flutter:
sdk: flutter
Save pubspec.yaml, and run flutter pub get to get the packages.
Setting up Your Flame Game Loop
The first step in creating your game is to make a Flame game loop. The game loop is the core component, the pulsing heart of your game. You’ll create and manage all your game components from here.
Open your lib folder, and create a file called forge2d_game_world.dart. Then, add a new class named Forge2dGameWorld
to this file. Your game will extend the base Forge2D game widget Forge2DGame
:
import 'package:flame_forge2d/flame_forge2d.dart';
class Forge2dGameWorld extends Forge2DGame {
@override
Future<void> onLoad() async {
// empty
}
}
FlameGame
class to get the Flame game loop and other core Flame properties and behaviors. A Forge2D game similarly extends Forge2DGame
. Forge2DGame
extends FlameGame
to provide Forge2D features in addition to those in FlameGame
for your game.
Next, open main_game_page.dart, and add these two imports with the other import statement at the top of the file:
import 'package:flame/game.dart';
import '../forge2d_game_world.dart';
Then, in the same file, create an instance of your new game loop class, replacing the comment // TODO: Create instance of Forge2dGameWorld here
.
final forge2dGameWorld = Forge2dGameWorld();
build
method will cause your game to rebuild every time the Flutter tree gets rebuilt, which usually is more often than you’d like.
Now, replace the Center
widget below the comment // TODO: Replace Center widget with GameWidget
with a GameWidget
and your forge2dGameWorld
instance:
child: GameWidget(
game: forge2dGameWorld,
),
Build and run your project. Now, you’ll see the familiar green border around a black rectangle, but the text is gone. The centered Text
widget has been replaced with your Flame GameWidget
, waiting for you to add game components.