How To Create A Simple 2D iPhone Game with OpenGL ES 2.0 and GLKit – Part 1
This is a blog post by site administrator Ray Wenderlich, an independent software developer and gamer. There are a lot of great tutorials out there on OpenGL ES 2.0, but they usually stop after drawing a rotating cube on the screen. How to take that rotating box and turn it into a full game is […] By Ray Wenderlich.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
How To Create A Simple 2D iPhone Game with OpenGL ES 2.0 and GLKit – Part 1
35 mins
This is a blog post by site administrator Ray Wenderlich, an independent software developer and gamer.
There are a lot of great tutorials out there on OpenGL ES 2.0, but they usually stop after drawing a rotating cube on the screen.
How to take that rotating box and turn it into a full game is usually left as an exercise to the poor reader. But how do you create classes for sprites, move them around, add your game logic, and handle scene management?
That, my friends, is where this tutorial series comes in! In this tutorial series we’re going to take the simple 2D “pew-pew ninja” game from our beginner Cocos2D tutorial and implement it completely in OpenGL ES 2.0, with GLKit!
I’ve tried to make this tutorial series as similar as possible to the above Cocos2D tutorial so you can compare the two to see the differences in implementation if you are curious.
The goal of this tutorial series is to keep things as simple as possible, and walk you through the process step by step. By the end, you’ll have a basic starting point you can use for your own 2D game engine!
Before reading this tutorial series, I recommend reading the Beginning OpenGL ES 2.0 with GLKit tutorial series. It’s also helpful (but not necessary) to read the Intermediate OpenGL ES 2.0 with GLKit chapter from iOS 5 by Tutorials.
Once you’ve read that, keep reading to make a simple 2D game for the iPhone – the hardcore way! :]
Why OpenGL ES 2.0 and GLKit?
For the skeptics out there, let’s discuss why you might want to make a game engine with OpenGL ES 2.0 and GLKit in the first place.
If you’re already convinced that this is what you wnat to do, feel free to skip this section and go straight to “Getting Started” :]
Why Use OpenGL ES?
Open GL ES is the lowest level graphics API on iOS. It interacts directly with the graphics card and is very powerful, so is often used to make games and highly visual apps.
The only problem with OpenGL is that it has a notoriously large learning curve and you have to write a lot of engine code to get a simple game working. Because of this, many programmers prefer to use a game engine instead, such as Cocos2D, Corona, or Unity.
Under the hood, all of these engines use OpenGL ES – they just hide the lower level details from you to make things simpler.
Although these game engines are powerful and can save you a lot of time, it’s still fun, and a good learning experience, to practice building a game engine yourself – using raw OpenGL ES.
You’ll learn a ton about game programming and OpenGL ES in the process, and better understand how to accomplish the effects you want in your games – whether you’re using your own engine, or one written by a third party!
Why Use OpenGL ES 2.0?
I’ve already discussed the difference between OpenGL ES 1.0 and OpenGL ES 2.0 in the Beginnng OpenGL ES 2.0 with GLKit tutorial series.
But as a quick reminder, OpenGL ES 2.0 is the way of the future, and you can make cooler effects with OpenGL ES 2.0 because it has shader support.
Since most modern devices supports OpenGL ES 2.0 now, for new apps I’d recommend going straight to OpenGL ES 2.0.
And for beginners, I’d also recommend you go straight to learning OpenGL ES 2.0 and not even bother with OpenGL ES 1.0 (or trying to learn them both at once). This tutorial (and the others on the site) don’t assume you have any prior OpenGL ES 1.0 experience.
Why Use GLKit?
GLKit is a set of APIs that makes working with OpenGL ES on iOS much easier than it used to be.
“But wait a minute!”, you might be thinking, “I thought we were trying to go as low level as possible!”
That’s true, but using GLKit doesn’t hurt you at all (using it doesn’t make you lose anything form the learning experience), but it does help you a ton.
GLKit saves you from writing some extremely boring (and easy to get wrong) code regarding setting up a basic display, creating basic shaders, performing vector/matrix math, and loading textures.
The number of boilerplate lines of code it takes to get started will be reduced significantly, and you can keep the focus on the game engine itself.
Bad-Ass Challenge: GLKit Setup
How bad ass do you feel today?
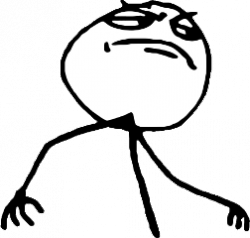
How bad ass do you feel today?
To get started, we’re going to create a simple project using OpenGL ES 2.0 and GLKit that just renders a green screen. Rather than using the “OpenGL Game” template, we’re going to do this “from scratch” so you understand how everything fits together better.
This will be review if you’ve gone through the Beginning OpenGL ES 2.0 with GLKit tutorial series.
So if you’ve read that tutorial series, feel comfortable with the material, and are feeling particularly bad-ass, see if you can complete this challenge without reading the step-by-step instructions below:
- Starting with the “Empty Application” template, create a simple OpenGL ES 2.0/GLKit project that renders the screen green. It should use ARC, a Storyboard, a GLKViewController and a GLKView.
If you haven’t read that tutorial or aren’t feeling particularly bad-ass today, keep reading for step-by-step instructions :]
Getting Started
Open Xcode, and create a new project with the iOS\Application\Empty Application template. Enter SimpleGLKitGame for the Product Name, SGG (for Simple GLKit Game) for the Class Prefix, and iPhone for the Device Family. Make sure Use Automatic Reference Counting is selected, and click Next.
First of all, we want this game to run in landscape only, so select your project in the Project Navigator and select your SimpleGLKitGame target. In the Summary tab’s Supported Device Orientations section, unselect the Portrait orientation as follows:
Second, we want to use OpenGL ES 2.0 and GLKit in this project, so we need to add some frameworks. Still with your project and target selected, select Build Phases, Expand the Link Binary With Libraries section, and click the Plus button. From the drop-down list, select the following frameworks and click Add:
- QuartzCore.framework
- OpenGLES.framework
- GLKit.framework
Next, let’s add a Storyboard to the project. Create a new file with the iOS\User Interface\Storyboard template, select iPhone for the Device Family, and name it MainStoryboard.storyboard.
Open MainStoryboard.storyboard, and drag a GLKit View Controller onto the storyboard. Since it’s your first view controller, Xcode will automatically set it up as the initial view controller. You know this because there’s an arrow to the left of the view controller, and “Is Initial View Controller” is checked.
We’re going to need to add some custom code to the view controller, so let’s create a subclass. Create a new file with the iOS\Cocoa Touch\UIViewController subclass template. Enter SGGViewController for the Class, GLKViewController for the Subclass, make sure both checkboxes are unchecked, click Next, and click Create.
To make the compiler happy, add the following to the top of SGGViewController.h:
#import <GLKit/GLKit.h>
Now open MainStoryboard.storyboard again. Select your view controller, and in the Identity Inspector set the Class to SGGViewController.
Now time for the code! Open up SGGViewController.m and replace the contents with the following:
#import "SGGViewController.h"
@interface SGGViewController ()
@property (strong, nonatomic) EAGLContext *context;
@end
@implementation SGGViewController
@synthesize context = _context;
- (void)viewDidLoad
{
[super viewDidLoad];
self.context = [[EAGLContext alloc] initWithAPI:kEAGLRenderingAPIOpenGLES2];
if (!self.context) {
NSLog(@"Failed to create ES context");
}
GLKView *view = (GLKView *)self.view;
view.context = self.context;
[EAGLContext setCurrentContext:self.context];
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return UIInterfaceOrientationIsLandscape(interfaceOrientation);
}
#pragma mark - GLKViewDelegate
- (void)glkView:(GLKView *)view drawInRect:(CGRect)rect {
glClearColor(0, 104.0/255.0, 55.0/255.0, 1.0);
glClear(GL_COLOR_BUFFER_BIT);
}
- (void)update {
}
@end
This is a bare bones implementation of a GLKViewController subclass. When the view loads it creates an OpenGL ES 2.0 context that it will use for further drawing, and associated it with the view. It also implements glkView:drawInRect to clear the screen to a green color. For review on this, check the previous tutorial.
One final step – we need to set up our project to use the storyboard we created. select your project in the Project Navigator and select your SimpleGLKitGame target. In the Summary Tab, set the Main Storyboard to MainStoryboard.storyboard.
Finally, open SGGAppDelegate.m and replace application:didFinishLaunchingWithOptions with the following (which will allow the main window to be created from the Storyboard rather than programatically):
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
return YES;
}
Compile and run, and enjoy your beautiful green screen! :]