iOS 7 Best Practices; A Weather App Case Study: Part 1/2
Learn various iOS 7 best practices in this 2-part tutorial series. You’ll master the theory and then practice by making a functional, beautiful weather app. By Ryan Nystrom.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
iOS 7 Best Practices; A Weather App Case Study: Part 1/2
30 mins
Finished Weather App
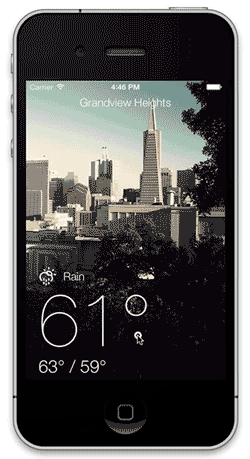
Finished Weather App
Every developer has their own ideas as to the best way to create top-notch iOS apps. Some developers take advantage of Auto-Layout, some like to create all of their graphics in code, and some developers even like to code in Vim!
With the recent release of iOS 7 and Xcode 5, I thought it would be a great time to provide a case study using a unique set of approaches and tools to create a basic weather app; you can look upon these as my recommended iOS 7 best practices. The first stepping-stone to iOS development used to be building a Todo List app. However, as iOS has matured new iOS developers are expected to be proficient in modern techniques such as data management and consuming web requests.
In this 2-part tutorial series, you’ll explore how to create your own apps using the following tools and techniques:
- Cocoapods
- Manual layout in code
- ReactiveCocoa
- OpenWeatherMap
This tutorial is designed for intermediate-level developers who know the basics, but haven’t moved into too many advanced topics. This tutorial is also a great start for anyone wanting to explore Functional Programming in Objective-C.
Getting Started
Fire up Xcode and go to File\New\Project. Select Application\Empty Application. Name your project SimpleWeather, click Next, select a directory to save your project, and then click Create.
You now have your base project set up. The next step is to set up your third-party tools — but first make sure you close Xcode so it won’t interfere with the next steps.
Cocoapods
You’ll be using Cocoapods to take care of downloading the code, adding files to your Xcode project, and configuring any project settings that those projects require. Let’s first cover what projects you’ll be using.
Mantle
Mantle is a project by the Github team that helps remove all of the boilerplate code that Objective-C requires to turn JSON data into NSObject subclasses. Mantle also does value transformation, which is a fancy way to turn JSON primitive values (strings, ints, floats) into complex values like NSDate, NSURL, or even custom classes.
LBBlurredImage
LBBlurredImage is a simple project that extends UIImageView and makes blurring images a breeze. You’ll be creating a fancy blur with just a single line of code. If you’re interested in seeing how the blur works, check out the source code.
TSMessages
TSMessages is another wonderfully simple library that takes care of displaying overlay alerts and notifications. When presenting error messages that don’t directly impact the user, it’s better to present an overlay instead of a modal view (like UIAlertView) so that you irritate the user as little as possible.
You’ll use TSMessages when a network connection is lost or the API encounters some other error. If something were to go wrong, you’d see an overlay like the one below:
ReactiveCocoa
The last library you’ll use is ReactiveCocoa, also made by the Github team. ReactiveCocoa brings functional programming to Objective-C by following patterns introduced by Reactive Extensions in .NET. You’ll be spending a good portion of your time in Part 2 implementing ReactiveCocoa.
Setting Up Your Cocoapods Libraries
To set up your Cocoapods libraries, first ensure that you have Cocoapods installed. To do that, open the Terminal application, type the following, and hit Enter.
which pod
You should see something similar to the following output:
/usr/bin/pod
Depending on how you manage your Ruby gems, for instance if you use rbenv or RVM, then your path may differ.
If the terminal simply returns to the prompt, or states pod not found
, Cocoapods isn’t installed on your machine; check out our tutorial on Cocoapods for installation instructions. It’s also a great resource if you just want to learn more about Cocoapods.
Podfiles are used to tell Cocoapods which Pods, or open-source projects, to import.
To create your first Cocoapod, first use the cd command in Terminal to navigate to the the folder where you saved your Xcode project. Launch the Pico editor by entering the pico command in Terminal.
Once pico has opened, paste in the following lines:
platform :ios, '7.0'
pod 'Mantle'
pod 'LBBlurredImage'
pod 'TSMessages'
pod 'ReactiveCocoa'
This file does two things:
- It tells Cocoapods which platform and which version you’re targeting. Here you’re targeting iOS 7.0.
- It gives Cocoapods a list of all the projects you want to import and set up.
To save your file, press Control-O, name the file Podfile and hit Enter. Now press Control-X to exit Pico.
To install the four Pods noted in your Podfile, type the following into Terminal and hit Enter.
pod install
Be patient — it could take a minute or two for pod to finish installing the various packages. Your Terminal output should look like the following:
You should see output like this:
$ pod install
Analyzing dependencies
CocoaPods 0.28.0 is available.
Downloading dependencies
Installing HexColors (2.2.1)
Installing LBBlurredImage (0.1.0)
Installing Mantle (1.3.1)
Installing ReactiveCocoa (2.1.7)
Installing TSMessages (0.9.4)
Generating Pods project
Integrating client project
[!] From now on use `SimpleWeather.xcworkspace`.
Cocoapods will create a bunch of new files in your project directory; however, the only one that you’ll be concerned about is SimpleWeather.xcworkspace.
Open SimpleWeather.xcworkspace in Xcode. Take a look at your project setup; there’s now a Pods
project in the workspace, as well as folders for each of the libraries you imported in the Pods folder, like so:
Make sure you have the SimpleWeather project selected, as shown below:
Build and run your app to make sure everything is working properly:
It doesn’t look like much right now, but you’ll add some content shortly.
Creating Your Main View Controller
Although the app looks complex, it will be powered by a single view controller. You’ll add that now.
With the SimpleWeather project selected, click File\New\File and select Cocoa Touch\Objective-C class. Name your class WXController and make it a subclass of UIViewController.
Make sure that both Targeted for iPad and With XIB for user interface are both unchecked, as shown below:
Open WXController.m and replace the boilerplate -viewDidLoad
method with the following:
- (void)viewDidLoad {
[super viewDidLoad];
// Remove this later
self.view.backgroundColor = [UIColor redColor];
}
Now open AppDelegate.m and import the following two classes:
#import "WXController.h"
#import <TSMessage.h>
Sharp-eyed readers will note that WXController
is imported with quotes, while TSMessage
is imported with angle brackets. What gives?
Look back to when you created the Podfile; you imported TSMessage
with Cocoapods. Cocoapods created the TSMessage Pod project and added it to your workspace. Since you’re importing from other projects in the workspace, you use angle brackets instead of quotes.
Replace the contents of -application:didFinishLaunchingWithOptions:
with:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
// 1
self.window.rootViewController = [[WXController alloc] init];
self.window.backgroundColor = [UIColor whiteColor];
[self.window makeKeyAndVisible];
// 2
[TSMessage setDefaultViewController: self.window.rootViewController];
return YES;
}
Walking through the numbered comments, you’ll see the following:
- Initialize and set the
WXController
instance as the application’s root view controller. Usually this controller is aUINavigationController
orUITabBarController
, but in this case you’re using a single instance ofWXController
. - Set the default view controller to display your TSMessages. By doing this, you won’t need to manually specify which controller to use to display alerts.
Build and run to see your new view controller in action:
The status bar is a little difficult to read against the red background. Fortunately, there’s an easy way to make the status bar a lot more legible.
There’s a new API in UIViewController in iOS 7 to control the appearance of the status bar. Open WXController and add the following code directly below -viewDidLoad
:
- (UIStatusBarStyle)preferredStatusBarStyle {
return UIStatusBarStyleLightContent;
}
Build and run again and you’ll see the following change to the status bar: