Showing an Empty Search Result
Right now, if your search produces no results, the screen will be blank. But it would be nicer to give the user some feedback instead of a blank screen. So, you'll implement a screen that will tell the user that their search came up empty.
First, add the following Composable at the end of WordlistUi.kt:
@Composable
private fun LazyItemScope.EmptyContent() {
Box(
modifier = Modifier.fillParentMaxSize(),
contentAlignment = Alignment.Center,
) {
Text(text = "No words")
}
}
This is a simple Composable that shows a Text
. You'll use it when there are no search results. The Composable extends from LazyItemScope
. This means you can use fillParentMaxSize
instead of fillMaxSize
. Doing so guarantees that the layout fills the size of the LazyColumn
.
Then, in the LazyColumn
in WordsContent
, call item
if there are no items. Inside the bottom of the LazyColumn
, use EmptyContent
to show an empty message:
if(items.itemCount == 0) {
item { EmptyContent() }
}
Build and run. Now, there's a screen that clearly shows the user there are no results for their search.
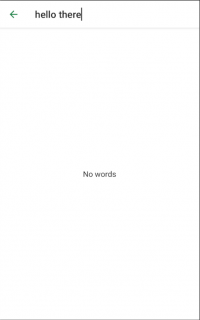
Finally, you've finished your app! Your users can look up the definition of English words. Your app will help people learn English and win at Scrabble. :]
Where to Go From Here?
You can download the final project by clicking the Download Materials button at the top or bottom of the tutorial.
By now, you see how easy it is to create declarative user interfaces with Compose and how the repository pattern complements this design.
If you want to learn more about Compose, check out the official tutorial and documentation from Google or our very own JetPack Compose by Tutorials.
If you want to learn what else is possible with Room, start with this tutorial about Data Persistence With Room or the official documentation, Save Data in a Local Database Using Room.
As a casual challenge, you can also try to extend the sample app's functionality so that your users can save their favorite words.
We hope you enjoyed playing with Compose and the repository pattern. If you have any questions, comments or ideas for things you'd like to see done with Compose, please join the forum discussion below!