How To Make a Custom Control Tutorial: A Reusable Slider
Controls are the bread-and-butter of iOS apps. There are many provided in UIKit but this tutorial shows you how to make a custom control in Swift. By Mikael Konutgan.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
How To Make a Custom Control Tutorial: A Reusable Slider
40 mins
Learn how to make a custom control like a slider that has two thumbs
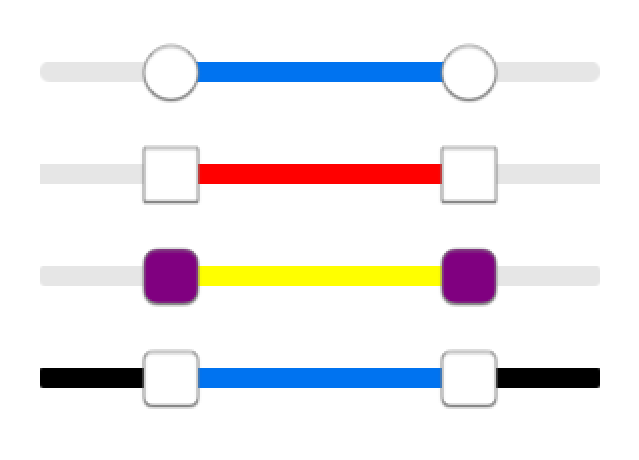
Learn how to make a custom control like a slider that has two thumbs
Update 12/8/14: Updated for Xcode 6.1.1.
Update note: This tutorial was updated for iOS 8 and Swift by Mikael Konutgan, checked against Xcode 6 beta 7. Original post by Tutorial Team member Colin Eberhardt.
User interface controls are one of the most important building blocks of any application. They serve as the graphical components that allow your users to view and interact with your application. Apple supplies a set of controls, such as UITextField
, UIButton
and UISwitch
. Armed with this toolbox of pre-existing controls, you can create a great variety of user interfaces.
However, sometimes you need to do something just a little bit different; something that the stock controls can’t handle quite the way you want.
Custom controls are nothing more than controls that you have created yourself; that is, controls that do not come with the UIKit framework. Custom controls, just like standard controls, should be generic and versatile. As a result, you’ll find there is an active and vibrant community of developers who love to share their custom control creations.
In this tutorial, you will implement your very own RangeSlider custom control. This control is like a double-ended slider, where you can pick both a minimum and maximum value. You’ll touch on such concepts as extending existing controls, designing and implementing your control’s API, and even how to share your new control with the development community.
Time to start customizing!
Getting Started
Say you’re developing an application for searching property-for-sale listings. This fictional application allows the user to filter search results so they fall within a certain price range.
You could provide an interface which presents the user with a pair of UISlider
controls, one for setting the maximum price and one for setting the minimum price. However, this interface doesn’t really help the user visualize the price range. It would be much better to present a single slider with two thumbs to indicate the high and low price range they are searching for.
You could build this range slider by subclassing UIView
and creating a bespoke view for visualizing price ranges. That would be fine for the context of your app — but it would be a struggle to port it to other apps.
It’s a much better idea to make this new component as generic as possible, so that you can reuse it in any context where it’s appropriate. This is the very essence of custom controls.
Fire up Xcode. Go to File/New/Project, select the iOS/Application/Single View Application template and click Next. On the next screen, enter CustomSliderExample as the product name, choose your desired Organization Name and Organization Identifier, then make sure that Swift is selected as the language, iPhone is selected as the Device and that Use Core Data is not selected.
Finally choose a place to save the project and click Create.
The first decision you need to make when creating a custom control is which existing class to subclass, or extend, in order to make your new control.
Your class must be a UIView
subclass in order for it be available in the application’s UI.
If you check Apple’s UIKit
reference, you’ll see that a number of the framework controls such as UILabel
and UIWebView
subclass UIView
directly. However, there are a handful, such as UIButton
and UISwitch
which subclass UIControl
, as shown in the hierarchy below:
UIControl
implements the target-action pattern, which is a mechanism for notifying subscribers of changes. UIControl
also has a few properties that relate to control state. You’ll be using the target-action pattern in this custom control, so UIControl
will serve as a great starting point.
Right-click the CustomSliderExample group in the Project Navigator and select New File…, then select the iOS/Source/Cocoa Touch Class template and click Next. Call the class RangeSlider, enter UIControl
into the Subclass of field and make sure the language is Swift. Click Next and then Create to choose the default location to store the file associated with this new class.
Although writing code is pretty awesome, you probably want to see your control rendered on the screen to measure your progress! Before you write any code for your control, you should add your control to the view controller so that you can watch the evolution of the control.
Open up ViewController.swift and replace its contents with the following:
import UIKit
class ViewController: UIViewController {
let rangeSlider = RangeSlider(frame: CGRectZero)
override func viewDidLoad() {
super.viewDidLoad()
rangeSlider.backgroundColor = UIColor.redColor()
view.addSubview(rangeSlider)
}
override func viewDidLayoutSubviews() {
let margin: CGFloat = 20.0
let width = view.bounds.width - 2.0 * margin
rangeSlider.frame = CGRect(x: margin, y: margin + topLayoutGuide.length,
width: width, height: 31.0)
}
}
The above code simply creates an instance of your all-new control in the given frame and adds it to the view. The control background color has been set to red so that it will be visible against the app’s background. If you didn’t set the control’s background to red, then the control would be clear — and you’d be wondering where your control went! :]
Build and run your app; you should see something very similar to the following:
Before you add the visual elements to your control, you’ll need a few properties to keep track of the various pieces of information that are stored in your control. This will form the start of your control’s Application Programming Interface, or API for short.