What’s New in Android Studio 3
Android Studio 3 was recently released – take a quick tour of what’s new! By Aldo Olivares.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
At I/O 2017, Google announced first-party support of the Kotlin programming language for Android Development, with Kotlin tools built right-in to an upcoming version of Android Studio.
Almost every Android developer around the world was excited for this change. Many have wanted an alternative to Java for several reasons, but until now had to use external plugins to achieve such a task.
On October 27, 2017, Android Studio 3.0 went to a stable version and many Android developers began to develop completely in Kotlin. We at raywenderlich.com are no exception, and most of our Android tutorials are now written in this great programming language.
But Kotlin support is only one of the changes in Android Studio 3. In this tutorial, you will learn about some of the other improvements that Android Studio 3 has to offer.
You will also learn about some of the best movies ever made thanks to our sample app: Color Movies.
Grab some popcorn and let’s code!
Note: For this project, I assume that you know the basics of Android development with Kotlin. You should also know how to set up and run Android Studio and the Android Emulator. If you need some help with that, check out our Beginning Android Development with Kotlin tutorial.
Note: For this project, I assume that you know the basics of Android development with Kotlin. You should also know how to set up and run Android Studio and the Android Emulator. If you need some help with that, check out our Beginning Android Development with Kotlin tutorial.
Getting Started
To kick things off, start by downloading the materials for this tutorial (you can find a link at the top or bottom of the page). The sample project has everything you need to try the new features in Android Studio 3.
Launch Android Studio 3.0.1 or greater, and in the Welcome to Android Studio window select Import Project (Eclipse, ADT, etc).
Select the high-level project directory and click OK.
If you explore the project, you will find a folder with models, a folder for the API services, a Kotlin extension file, an activity and a layout file. It is not necessary that you analyze them in detail since you won’t be heavily editing these files. Instead, we are going to focus on the advantages that Android Studio 3.0 provides to work with them.
Build and Run the app on an emulator or device. You should see this:
The screen of our activity contains a button and some text. Don’t press the button just yet. First, you will need to obtain an API Key.
Obtaining the NY Times API Key
For this tutorial you will use the NY Times Open API, a popular web service for retrieving movie and book reviews.
Fortunately, getting an API Key is as easy as going to https://developer.nytimes.com/signup
Note: You will be asked to select an API. Choose Movie Reviews API.
Note: You will be asked to select an API. Choose Movie Reviews API.
Once you have acquired your API key, open the NYTimesApi.kt file under the models folder and replace the part that says <key> with your own.
@GET("svc/movies/v2/reviews/search.json?api-key=<key>")
fun getReviews(): Call<MovieResponse>
Build and Run:
Press the New Movie button to get an awesome movie suggestion:
Now we are ready to try all the new features in Android Studio 3! :]
Development
Kotlin
The newest and most anticipated feature is app development in Kotlin. One of the best parts of Kotlin is that this language is 100% interoperable with Java. Therefore, the most popular libraries that you know and love will work without any issue with the help of Gradle.
This project uses Retrofit, one of the most popular Android Networking libraries written in Java.
To see how libraries are implemented in Android Studio 3, open the build.gradle file of the app:
Android Studio 3 Gradle configuration has changed since the last version, due to changes in the Android Studio Gradle plugin version 3.0.0+. Among other changes, we now typically use implementation instead of compile:
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation"org.jetbrains.kotlin:kotlin-stdlib-jre7:$kotlin_version"
implementation 'com.android.support:appcompat-v7:26.1.0'
implementation 'com.android.support.constraint:constraint-layout:1.0.2'
implementation 'com.squareup.retrofit2:converter-moshi:2.3.0'
implementation "com.android.support:design:$supportVersion"
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.1'
androidTestImplementation
}
Also, you no longer need to specify the Android build tools version in build.gradle. The plugin will use the minimum required version by default. So, you can now omit the android.buildToolsVersion
property.
Note: We are not going to cover all the changes in the Gradle plugin because that would be beyond the scope of this tutorial. If you want more information, you can check out the official documentation or our Gradle Tutorial for Android. :]
Note: We are not going to cover all the changes in the Gradle plugin because that would be beyond the scope of this tutorial. If you want more information, you can check out the official documentation or our Gradle Tutorial for Android. :]
Navigate to the models package and open the Link.kt file.
This class consists of a single statement thanks to the power of the data classes in Kotlin:
data class Link(val type: String,
val url: String,
val suggested_link_text: String)
To give you an idea of how much code you were saved, this would be the Java counterpart:
public class Link {
private String type;
private String url;
private String suggested_link_text;
public Link(String type, String url, String suggested_link_text) {
this.type = type;
this.url = url;
this.suggested_link_text = suggested_link_text;
}
public String getType() {
return type;
}
public String getUrl() {
return url;
}
public String getSuggested_link_text() {
return suggested_link_text;
}
}
Cool right?
You are not going to learn all ins and outs of Android Development with Kotlin in this project. I invite you to explore our Kotlin for Android tutorial if you want to learn more.
Custom and Downloadable Fonts
Beginning with Android Studio 3, we can let the system request fonts from a provider application instead of bundling them into our APKs, or let our APK download fonts. Custom font support in your app is provided on older Android devices via support library 26 and above.
Custom and downloadable fonts have several benefits including:
- Android Studio 3 design editor support
- Increased app installation success rate
- Reduced APK size
- Less memory and data usage since they are only fetched over the network when needed.
To try this new feature you are going to modify the Color Movies app to use a custom font from Google Fonts.
Open activity_main.xml in the res/layout folder.
In the Android Studio design editor preview, you will notice that the font is the usual sans serif:
Select the TextView that displays the movie review:
Go to the fontFamily dropdown menu, scroll down and select More Fonts…
A menu will show up that lets you select between Android’s native fonts or custom Downloadable fonts from Google Fonts:
Look for the Aldrich font either by scrolling down or by using the search field, and select it:
To use the custom font we have two options: Create downloadable font or Add font to the project.
Downloadable fonts are usually the best choice since they let Android download it only when necessary, saving memory and mobile data usage.
Make sure that Create downloadable font is selected and click OK.
Your layout preview should now look like this:
There is also a new file under your resfolder:
The aldrich.xml file contains all the information needed by Android devices to download your font when needed.
Now you need to add the same font to the remaining TextViews. Fortunately, now that you have added it to your res folder all you have to do is select it in the fontFamily dropdown menu:
The little clip-shaped icon indicates that it is a downloadable font instead of a native font.
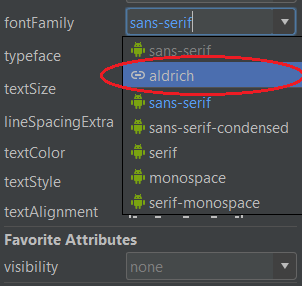
The little clip-shaped icon indicates that it is a downloadable font instead of a native font.
After you have finished changing the font for both TextViews your layout should look like this:
Build and Run the app:
Now your app has a nice custom font that Android will automatically download when needed!
Click on New Movie to see your new font in action:
Wow, Maze Runner has a great review! We should head to the movies right now! :]
There is a lot more to learn about custom fonts in Android. If you want to learn more, please make sure to check out our Custom and Downloadable Fonts tutorial.